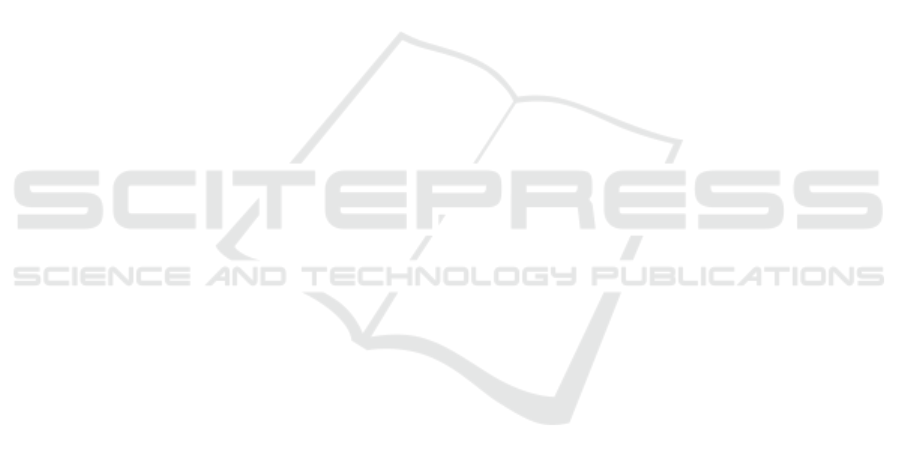
and passing the control back to the host application to
prevent the enclave from executing forever.
Binary sizes of the enclave applications for vari-
ous optimization levels are presented in Table 2. All
binaries were stripped from symbols and debug infor-
mation to have result comparable to production code.
The C and Rust programs are compiled using differ-
ent compilers. Thus, the optimizations performed for
them might be different even for identical optimiza-
tion levels. The results show the Rust applications are
around 2-3 times larger than their C equivalents. One
of the reasons for this is that the Keystone SDK only
contains a very minimal C layer, while the Rust SDK
contains higher-level abstractions and more checks
for the incoming and outgoing data. In addition, the
linker script for the applications is different, causing
some program sections to be a bit larger.
Rust SDK’s ecall performance is represented in
Table 3. Measurements were made for the enclave
application, i.e., instructions to serve single call, and
the host application, i.e., instructions to make a sin-
gle call. The x column shows the ratio of the instruc-
tion count to that of an ocall with the same amount of
payload data. In the host application, the instruction
count is measured from the thread initiating the ecall,
thus thread synchronization is included. The original
C/C++ SDK doesn’t support ecalls, thus these mea-
surements are only shown for the Rust SDK.
The results show that an emulated ecall is approx-
imately 2 to 6 times slower than an ocall. As men-
tioned in Section 3.4, both all the data needs to be
copied one extra time during emulation. Since on
larger payload sizes the number of measured instruc-
tions is dominated by the instructions needed to copy
the payload data, the performance is as expected.
5 DISCUSSION
The Rust SDK API for enclave applications is rather
minimalistic and Keystone Eyrie specific. To enhance
portability to other enclave architectures, a more stan-
dardized enclave API could be implemented on top
of our API. To our knowledge, no such API defini-
tion exists yet for the Rust language and most existing
standard APIs only define C or C++ bindings.
Most real-world enclave applications need func-
tionality that the Rust SDK does not yet offer, al-
though some of it can be included using existing Rust
libraries. One of the most important and commonly
used features is persistent secure storage that is sealed
to the underlying platform. Keystone derives data
sealing keys for each enclave, which the Rust API
also exposes to the enclave applications. However,
persistent storage must be implemented with the sup-
port of the host application or operating system to
store the encrypted data on the untrusted platform. As
the implementation is complex, it makes sense to pro-
vide a common API and implementation in the en-
clave library. Other generic functionality that could
be added into the API includes derivation of enclave
specific keys and other common cryptographic opera-
tions, such as data signing and verification.
One motivation for our research is to decrease vul-
nerabilities in security critical software. Using Rust,
or any other safe programming language, is not a sil-
ver bullet to increase security (Xu et al., 2021) or to
guarantee that the enclave interface or applications are
secure (Van Bulck et al., 2019). Safe programming
languages are only enablers for writing more secure
software. Nevertheless, the Rust language allows par-
ticular types of security issues, e.g., buffer overflows,
to potentially be detected more easily and earlier dur-
ing development. In addition, Rust language’s strict
type system and aliasing rules, applied also to mem-
ory and threads, enable stronger static analyses to be
used for security assurance.
Thorough security analysis and hardening of our
SDK is still work to be done before it can be claimed
to accomplish our intended goal. Van Bulck et al.
(Van Bulck et al., 2019) analyzed vulnerabilities in
input sanitization at the boundary of enclave appli-
cations and untrusted code in several TEEs. They
found several vulnerabilities in runtime implementa-
tions and at enclave ABI and API layer, some also in
Keystone. Their insight to enclave security issues and
proposed mitigations form a good starting point for
our future work to ensure security of the SDK.
We limited our work to the enclave and host ap-
plication APIs and their implementation. However,
security of the enclave application depends on many
layers, none of which can be overlooked. First, se-
curity of the enclave architecture, its ABI, and the
implementation, in this case the Keystone Security
Monitor, its driver for the untrusted OS, and the Eyrie
runtime functioning as the OS for the enclave appli-
cation. High-level security analysis of the Keystone
framework was presented by Lee et al. (Lee et al.,
2020). However, Keystone is still a research proto-
type and we are not aware if detailed security anal-
ysis and hardening of the implementation has been
performed. Second, security of the API used by the
applications and its implementation, i.e., in our case
the Rust SDK. As said, this is still work to be done.
Finally, security of the application built on top of the
APIs. It is impossible to anticipate how the applica-
tions are written, and thus, to prevent or mitigate all
the vulnerabilities in the runtime. Nevertheless, the
Towards a Rust SDK for Keystone Enclave Application Development
35