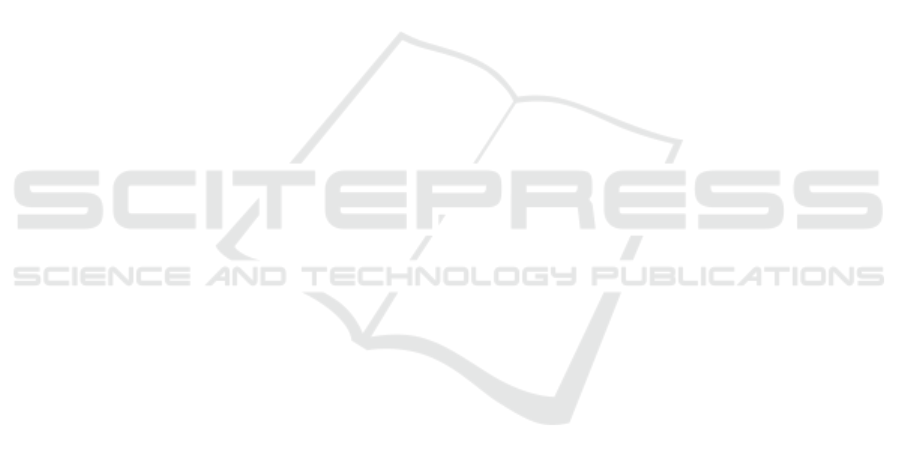
perform better optimizations and thus the execution
speed can be influenced. In statically compiled lan-
guages, this can be achieved with different guidelines,
however for JavaScript, such guidelines are not com-
mon.
Several guidelines for JavaScript have been pub-
lished addressing this topic (Wilton-Jones, 2006; Za-
kas, 2009a; Zakas, 2009b; Herczeg et al., 2009; Her-
czeg et al., 2012; Osmani, 2012), and most of them
overlap each other. One of them presents a con-
crete comparison of different web browsers on the
available JavaScript guidelines at the time (Herczeg
et al., 2012) . It reveals several correlations between
JavaScript engines and optimal guidelines. In this pa-
per, the legacy guidelines are re-examined and it is
measured how they perform in the current version of
the JavaScript engines. Additionally, with the evo-
lution of the JavaScript language some of the new
constructs are investigated and measured, resulting in
new guidelines and optimizations.
The rest of the paper is organized as follows. In
Section 2, the guidelines and the reasons for their im-
portance are discussed. The legacy guidelines are also
introduced, and some of the new language constructs
are presented. In Section 3, the results for the guide-
lines are discussed and the new guidelines are pre-
sented. In Section 4, the related works are presented.
Finally, the paper is concluded in Section 5.
2 GUIDELINES
Developers use several kind of guidelines in their
development processes: to keep the source code in
style, to satisfy naming conventions, to apply differ-
ent kinds of APIs, and to conform to such software
requirements as optimal performance or user experi-
ence. Although formating and structure related top-
ics are well known and analyzed by many in gen-
eral, e.g. (Fard and Mesbah, 2013), performance
related guidelines are a neglected area in scripting
languages. As we have mentioned, relatively few
researches and comparisons focusing on the perfor-
mance of JavaScript in terms of guidelines have been
done.
Before going forward, we are going to define what
a guideline is. A guideline is a code transforma-
tion which shows how to convert one source code
to another while solving a previously defined prob-
lem. Guidelines are not binding and are not enforced.
In this paper, this means that code transformation is
a similar code conversion action on JavaScript code
snippets which tries to improve the performance of
the container software.
We would like to note that the reason why we are
focusing on similar and not identical code transfor-
mation is that similar transformation does not limit
the usage of code structures and language features as
opposed to identical transformation which sometimes
impossible or can be very expensive. Of course, in
many cases it is possible to create an equivalent code
transformation which tries to address the same goal,
but in these cases the domain of the transformation
will be much narrower. Consequently, we will focus
on those similar code transformations which are about
to improve the performance.
2.1 Legacy Guidelines
In the previously published researches and blog posts
(Wilton-Jones, 2006; Zakas, 2009a; Zakas, 2009b;
Herczeg et al., 2009; Herczeg et al., 2012; Osmani,
2012) several guidelines were listed. Most of them
were created to speed up execution, but not all of
them concentrated on the JavaScript module itself.
Many suggestions and guidelines are about to im-
prove the performance of the Web browser instead
of the JavaScript engine itself. We are only focusing
on the performance topic of JavaScript engines. In
the upcoming sub-sections, we present the overview
of the legacy guidelines from the previously cited re-
searches.
• Using Local Variables: This guideline describes
that it is more fruitful to use local variables instead
of global ones. The reason behind this guideline
is very simple; try to reduce the visibility of a
variable. It is a common programming paradigm.
The architecture logic behind this is that a com-
plex lookup method is called each time the global
variable is accessed, and this lookup traverses the
whole scope chain every time.
• Using Global Static Data: There is an exception
to the previously defined Using Local Variables
guideline. This exception is when the developer
wants to introduce a large global static object. In
this case, if one is about to use the large static data
as a local variable it will be constructed every time
when the data is accessed. This guideline suggests
avoiding to create the same large object in every
usage even if it is a local data. It is better to use a
global one instead.
• Caching Object Members: It is a general use-case
in the development process to access a complex
object structure within a loop. In this case, it is
possible to save the object field lookup if it is
stored in a local variable. The reason for this is
similar to the explanation given at Using Local
Variables guideline.
ICSOFT 2018 - 13th International Conference on Software Technologies
398