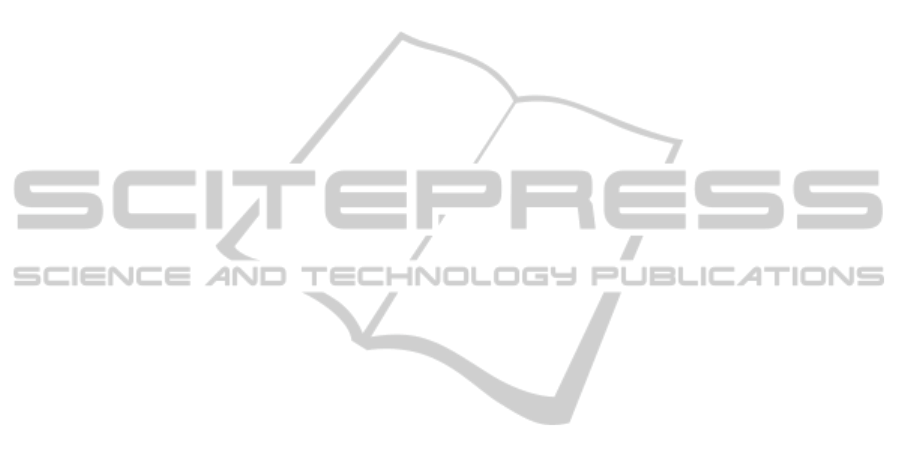
their functionality.
Reusability: A measure of how easy it is to reuse a
module in a different system. As a consequence of
low coupling, it should be easier to reuse a module
that was implemented in the past for a previous
system, because that module should be less
dependent on the rest of the system. Accordingly, it
should be easier to reuse the modules of the current
system in new future systems. As a consequence of
high cohesion, the functionality provided by a
module should be well-defined and complete,
making it more useful as a reusable component.
2 EVENT-DRIVEN APPROACH
Event-Driven Programming (EDP) can also be seen
as a tool for the Separation of Concerns. There is a
clear intention of reducing the coupling between the
modules that trigger the events and the modules that
handle these events. Ideally, the modules which are
triggering the events should not be aware of the
modules that will handle them. The modules
triggering the events should not be concerned of
how these events will be handled, or even if a
particular event will be handled at all. EDP also
helps to increase the cohesion of modules, since it
allows separating the business logic of handling the
event from the function that triggered it. Both the
event triggering module and the event handler
become more cohesive. Of course, there can be
several handlers for the same type of event, each one
with a very specialized way to handle it, what
further decreases coupling and increases cohesion.
Some systems are essentially event-driven, for
example Reactive Systems, in which their main
input from the external environment is in the form of
events, and in this case using EDP is a natural and
almost required design decision. Some systems are
implemented using EDP as a consequence of an
analysis and modelling approach, for example action
games, but in this case using EDP is an option and
they could be modelled in a different way. Finally,
some systems are hybrid, and they use EDP only to
implement a specific part of their functionality, for
example the Graphical User Interface, a Publish-
Subscribe subsystem (Eugster, 2003) or even a
simple Observer pattern (Gamma, 1995). In most of
these situations, the decision of using EDP is not a
consequence of its ability to separate concerns. Very
often it is simply the most convenient way to model
a system or some part of the system.
In this paper we claim that EDP should be
adopted as an alternative tool for the Separation of
Concerns. This means that when a software
developer is confronted with the problem of
reducing the coupling and increasing the cohesion of
a system, he should consider adopting an EDP
approach, based on the explicit definition of Events
and Event Handlers. Further yet, we believe that an
EDP approach has advantages and can provide
benefits that cannot be easily obtained with OOP or
AOP.
In the remaining sections of this paper we
describe a simple framework to support the
introduction of EDP in a system, we provide a brief
example of an application in which EDP is
effectively used to separate concerns, and we present
a more detailed comparison of the advantages and
disadvantages of EDP when compared to AOP.
2.1 The EventJ Framework
In order to analyze the efficacy of the event-driven
approach for the separation of concerns, we
implemented a framework in Java called EventJ.
The two main concepts in the EventJ framework are
the Events and their respective EventHandlers.
Events: An Event is an immutable object which has
state and may have functions that perform some
computation over this state. Events have type and
are organized in an inheritance hierarchy.
EventHandlers: An EventHandler is responsible for
executing some action in response to an Event. A
single EventHandler may subscribe to different
types of Events. If an EventHandler subscribes to an
Event type, it handles also all instances of its
subtypes. EventHandlers may be stateless or stateful.
An EventHandler may trigger Events itself.
EventHandlers receive Events asynchronously and
should not depend on the results of other
EventHandlers. Each EventHandler runs on a
separate thread, and manages its own queue of
Events.
EventDispatcher: The EventDispatcher is a
Singleton object (Gamma, 1995) which is
responsible for the propagation of all Events to the
appropriate EventHandlers. When an Event is
triggered anywhere in the system, it is initially
stored in the EventDispatcher’s central queue. Then,
according to the Event type, each Event is
asynchronously propagated to all the EventHandlers
that have subscribed to its type (or to its supertypes).
When an EventHandler starts its execution, the first
step is to call the EventDispatcher in order to
subscribe to all types of Events it intends to handle.
The EventDispatcher runs on a separate thread.
Of course, the appropriate usage of the EventJ
AnEvent-drivenApproachfortheSeparationofConcerns
123