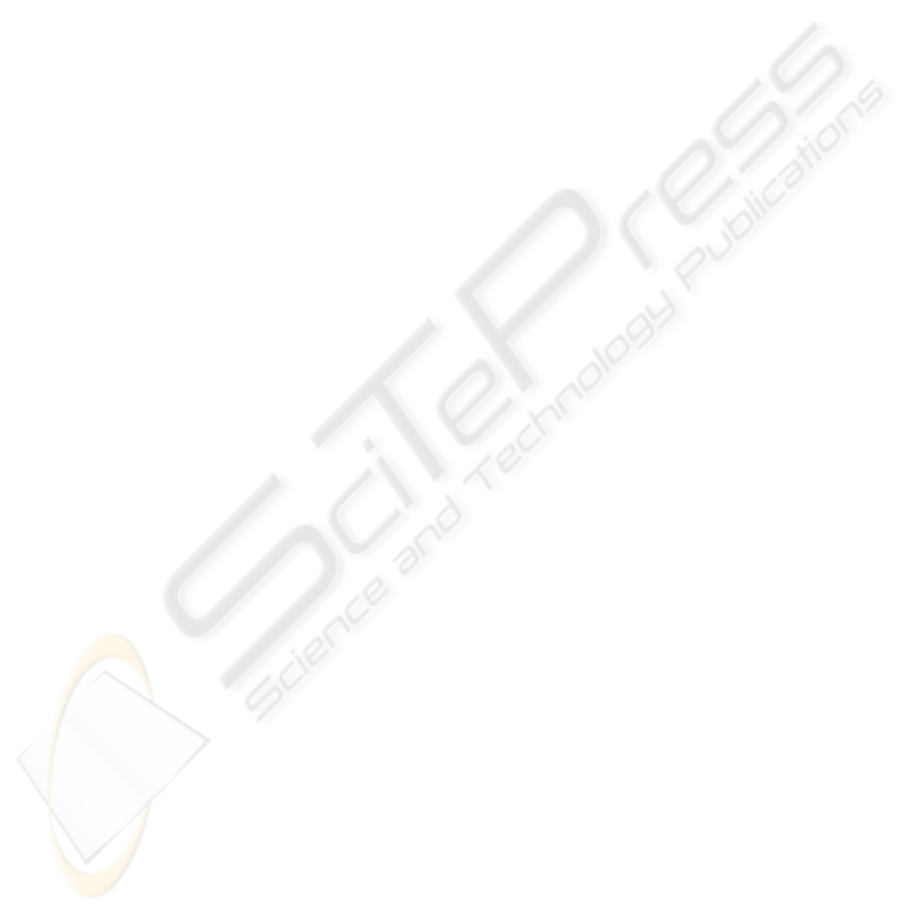
2. AspectJ Team, The. The AspectJ Programming Guide. 2003.
3. Balzer, S., Eugster, P., and Meyer, B. Can Aspects Implement Contracts? Proceedings of
Rapid Integration of Software Engineering techniques (RISE), Geneva, Switzerland, Sep-
tember 13-15, 2006.
4. Burdy, L., Cheon, Y., Cok, D., et al. An overview of JML tools and applications. Interna-
tional Journal on Software Tools for Technology Transfer, June 2005.
5. Duncan, A., and Hölzle, U. Adding Contracts to Java with Handshake. Technical report
TRCS98-32, December 8, 1998.
6. Ecma. Eiffel: Analysis, Design and Programming Language (2nd ed.), June 2006.
7. Findler, R., and Felleisen, M. Contract Soundness for Object-Oriented Languages. Confe-
rence on Object Oriented Programming Systems Languages and Applications (OOPSLA),
Florida, USA, 2001.
8. First Person Inc. Oak Language Specification. 1994.
9. Gosling, J., Joy, B., Steele, G., and Bracha, G. The Java Language Specification (3rd edi-
tion). Prentice-Hall, 2005.
10. Guerreiro, P. Simple Support for Design by Contract in C++, TOOLS USA 2001, Proceed-
ings, pages 24-34, IEEE, 2001.
11. Hoare, C. An Axiomatic Basis for Computer Programming. Communications of the ACM,
Vol. 12, No. 10, October 1969.
12. Karaorman, M., and Abercrombie, P. jContractor: Introducing Design-by-Contract to Java
Using Reflective Bytecode Instrumentation. Formal Methods in System Design, Vol. 27,
No. 3, November, 2005.
13. Kramer, R. iContract - the Java design by contract tool. 26th Technology of Object-
Oriented Languages and Systems (TOOLS), California, USA, 1998.
14. Laddad, R. AspectJ in Action: Pratical Aspect-Oriented Programming. Manning, 2003.
15. Lindholm, T., and Yellin, F. The Java Virtual Machine Specification. Prentice-Hall, 1999.
16. Liskov, B., and Wing, J. Family Values: A Behavioral Notion of Subtyping. Technical
report MIT/LCS/TR-562b, Carnegie Mellon University, July 16, 1993.
17. Meyer, B. Eiffel: The Language. Prentice-Hall, 1991.
18. Meyer, B. Object-Oriented Software Construction (2nd ed.). Prentice-Hall, 1997.
19. Mitchell, R., and McKim, J. Design by Contract, by Example. Addison-Wesley, 2002.
20. OMG Unified Modeling Language (UML) 2.0 OCL convenience document. 2005.
21. Plösh, R. Evaluation of Assertion Support for the Java Programming Language. Journal of
Object Technology, Vol. 1, No. 3, Special Issue: TOOLS USA 2002 proceedings.
22. Rieken, J. Design by Contract for Java - Revised (master thesis), Carl von Ossietzky Un-
iversität - Correct System Design Group, April 24th, 2007.
23. Sun Microsystems Java 2 Platform Standard Edition 5.0 API Specification. 2004.
24. Wampler, D. Contract4J for Design by Contract in Java: Designing Pattern-Like Protocols
and Aspect Interfaces. Industry Track at AOSD 2006, Bonn, Germany, March 22, 2006.
128