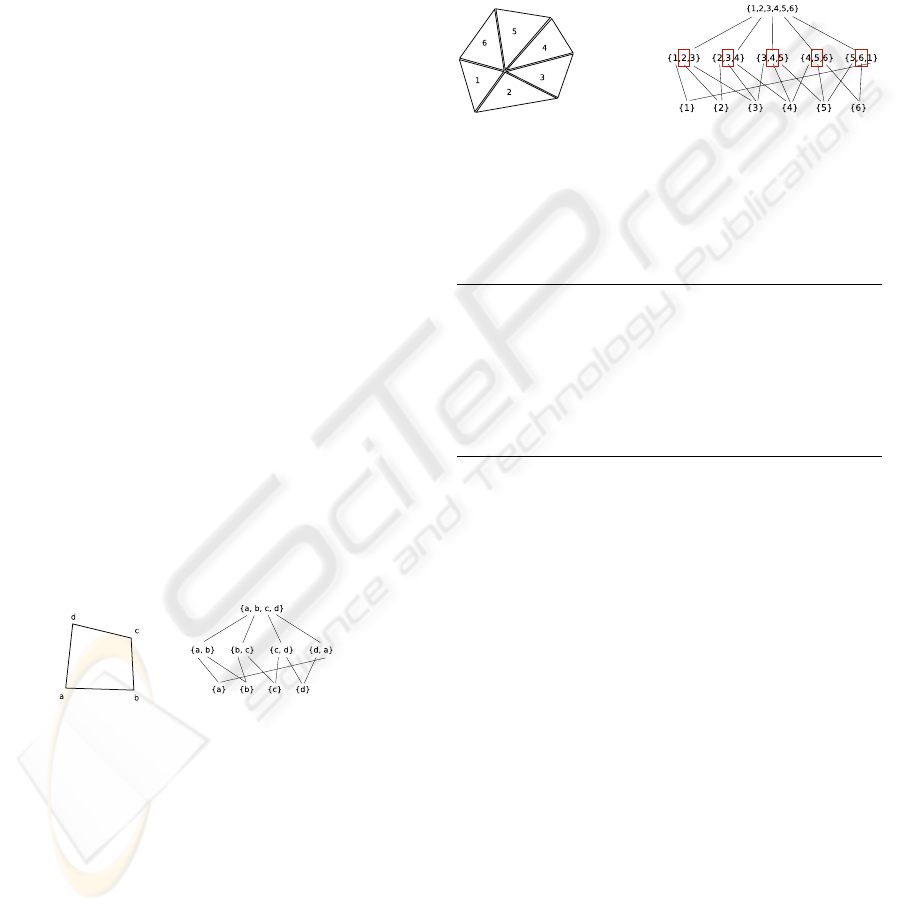
of the solution and the amount of affordable compu-
tational resources are best met by different simulation
methodologies.
2 THE GENERIC SCIENTIFIC
SIMULATION ENVIRONMENT
Our approach of transforming different methodolo-
gies into high performance applications is based on
the GSSE. It provides a domain specific embedded
language for mathematical notation as well as data
structures directly within C++, which greatly eases
the specification of formulae and provides the func-
tional dependencies inherent in the formulae.
Another important part of the environment is to
offer consistent interfaces by using concept based
generic programming to achieve interoperability be-
tween different library approaches. Furthermore, we
developed a consistent data structure interface for
all different types of data structures (STL (Austern,
1998), BGL (Siek et al., 2002), GrAL (Berti, 2002),
CGAL (Fabri, 2001)) based on algebraic topology
and poset theory (Heinzl et al., 2006b). With this in-
terface specification we can make use of several al-
ready available libraries within the GSSE. High in-
teroperability and code reuse can thereby be accom-
plished without incurring overhead.
Algebraic topology is used for the interface
and traversal specification further separating the cell
topology from the complex topology. Code complex-
ity is thereby reduced greatly, while at the same time
code reuse is increased. Figure 1 depicts the topolog-
ical properties of a cuboid cell and the correspond-
ing projection into a partially ordered set (poset).
Figure 1: Cell topology of a cuboid cell in two dimensions.
Using this poset it is possible to identify all inter-
dimensional objects such as edges and facets and their
relations to the cell. Therefore, traversal of all of
these different objects is completely determined by
this structure and the vertex on edge, vertex on cell,
and edge on cell traversal up to the dimension of the
cell can be derived automatically by the compiler it-
self without the need of user intervention.
In addition to the cell topology the complex topol-
ogy can be derived in order to best support a unique
characterization and orthogonal implementation. A
basic example is depicted in Figure 2, which presents
the structure of a three-dimensional cell complex.
Only locally neighboring cells can be traversed by this
data structure, which can be seen in the poset on the
right hand side. The number of elements in the meta-
cell’s subsets is bounded by a constant number, which
is unique for each type of data structure. The term
meta-cell is used to describe various subsets with a
common name, e.g., the singly linked list uses two
elements per meta-cell, later stated as
local(2)
and
called
forward
concept in the STL.
Figure 2: Complex topology of a three-dimensional simplex
cell complex.
In order to demonstrate the equivalence of our data
structure and the STL data structures a simple code
snippet is presented. The corresponding and required
typedef
definitions are omitted:
cell_type <0, simplex> cells_t;
complex_type <cells_t, global > complex_t;
long
data_t;
container_t <complex_t ,data_t > container;
// is equivalent to the STL type
std::vector<data_t> container;
Equivalence of Data Structures
The distinct areas of scientific computing yield
themselves differently well to implementations us-
ing one of the many programming techniques. In
several cases the demand for a specific program-
ming paradigm can be observed. We provide a short
overview of several programming paradigms and their
advantages:
• Object-oriented programming: is the close inter-
action of content and mechanisms related to the
particular content. Algorithms can not be speci-
fied independently of object structures.
• Functional programming: is inherently parallel
and side-effect free. However, most functional
programming languages suffer from great perfor-
mance shortcomings.
• Generic programming: is the glue between object-
oriented programming and functional program-
ming.
Many difficulties encountered in conventional pro-
gramming can be circumvented with the approach
of using the paradigms shown above appropriately
at the same time. Functional programming enables
SIMULATION METHODOLOGIES FOR SCIENTIFIC COMPUTING - Modern Application Design
271