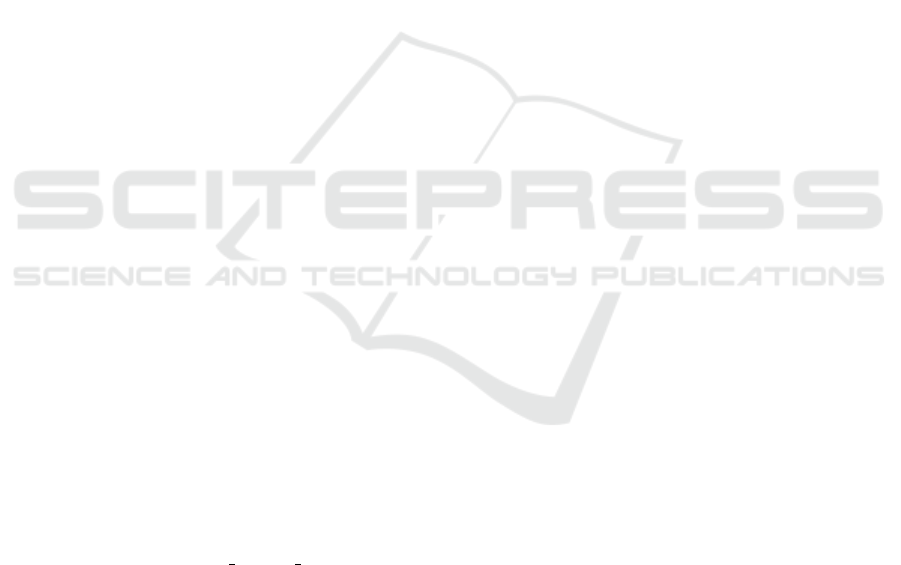
Baker, H. G. (1992). The treadmill: Real-time garbage col-
lection without motion sickness. ACM Sigplan No-
tices, 27(3):66–70.
Bannister, A. (2021). Substandard software costs
us economy $2tn through security flaws,
legacy systems, abandoned projects. URL:
https://portswigger.net/daily-swig/substandard-
software-costs-us-economy-2tn-through-security-
flaws-legacy-systems-abandoned-projects.
Barnett, M., Naumann, D. A., Schulte, W., and Sun, Q.
(2004). 99.44% pure: Useful abstractions in speci-
fications. In ECOOP workshop on formal techniques
for Java-like programs (FTfJP). Citeseer.
Bobrow, D. G. (1980). Managing reentrant structures using
reference counts. ACM Transactions on Programming
Languages and Systems (TOPLAS), 2(3):269–273.
Cheney, C. J. (1970). A nonrecursive list compacting algo-
rithm. Communications of the ACM, 13(11):677–678.
Cimpanu, C. (2019). Microsoft: 70 percent of all security
bugs are memory safety issues. URL: https://www. zd-
net. com/article/microsoft-70-percent-of-all-security-
bugs-are-memory-safety-issues.
Clark, D. W. (1979). Measurements of dynamic list struc-
ture use in lisp. IEEE Transactions on Software Engi-
neering, (1):51–59.
Clark, D. W. and Green, C. C. (1977). An empirical study
of list structure in lisp. Communications of the ACM,
20(2):78–87.
Cohen, J. and Nicolau, A. (1983). Comparison of com-
pacting algorithms for garbage collection. ACM
Transactions on Programming Languages and Sys-
tems (TOPLAS), 5(4):532–553.
cppreference (2012). C++ mutable. https://en.cppreference.
com/w/cpp/language/cv.
Dange, S. and Chatterjee, M. (2020). Iot botnet: the largest
threat to the iot network. In Data Communication and
Networks, pages 137–157. Springer.
Dawson, M., Burrell, D. N., Rahim, E., and Brewster, S.
(2010). Integrating software assurance into the soft-
ware development life cycle (sdlc). Journal of Infor-
mation Systems Technology and Planning, 3(6):49–
53.
Deutsch, L. P. and Bobrow, D. G. (1976). An efficient, in-
cremental, automatic garbage collector. Communica-
tions of the ACM, 19(9):522–526.
dlang.org (2012). Implicit qualifier conversions. dlang.org/
spec/const3.html#implicit qualifier conversions.
Fenichel, R. R. and Yochelson, J. C. (1969). A lisp garbage-
collector for virtual-memory computer systems. Com-
munications of the ACM, 12(11):611–612.
Goldberg, B. (1991). Tag-free garbage collection for
strongly typed programming languages. In Pro-
ceedings of the ACM SIGPLAN 1991 conference on
Programming language design and implementation,
pages 165–176.
Hayes, B. (1991). Using key object opportunism to col-
lect old objects. In Conference proceedings on Object-
oriented programming systems, languages, and appli-
cations, pages 33–46.
Jones, R., Hosking, A., and Moss, E. (2016). The garbage
collection handbook: the art of automatic memory
management. CRC Press.
Knuth, D. E. (1997). The art of computer program-
ming. volume 1: Fundamental algorithms. volume 2:
Seminumerical algorithms. Bull. Amer. Math. Soc.
Larson, R. G. (1977). Minimizing garbage collection as a
function of region size. SIAM Journal on Computing,
6(4):663–668.
Lee, P. (1991). Topics in Advanced Language Implementa-
tion. MIT Press.
McBeth, J. H. (1963). Letters to the editor: on the refer-
ence counter method. Communications of the ACM,
6(9):575.
McCarthy, J. (1960). Recursive functions of symbolic ex-
pressions and their computation by machine, part i.
Communications of the ACM, 3(4):184–195.
McMillen, D. (2021). Internet of threats: Iot bot-
nets drive surge in network attacks. URL:
https://securityintelligence.com/posts/internet-of-
threats-iot-botnets-network-attacks/.
Romanazzi, S. (2018). From manual memory manage-
ment to garbage collection. ResearchGate [On-
line]. Available from: https://doi. org/10.13140/RG,
2(22961.28006).
Ullrich, S. and de Moura, L. (2019). Counting immutable
beans: Reference counting optimized for purely func-
tional programming. In Proceedings of the 31st Sym-
posium on Implementation and Application of Func-
tional Languages, pages 1–12.
Wentworth, E. (1990). Pitfalls of conservative garbage
collection. Software: Practice and Experience,
20(7):719–727.
Whittaker, Z. (2016). Mirai botnet attackers are try-
ing to knock an entire country offline. URL:
https://www.zdnet.com/article/mirai-botnet-attack-
briefly-knocked-an-entire-country-offline/.
Wilson, P. R. (1992). Uniprocessor garbage collection tech-
niques. In International Workshop on Memory Man-
agement, pages 1–42. Springer.
ICSOFT 2022 - 17th International Conference on Software Technologies
306