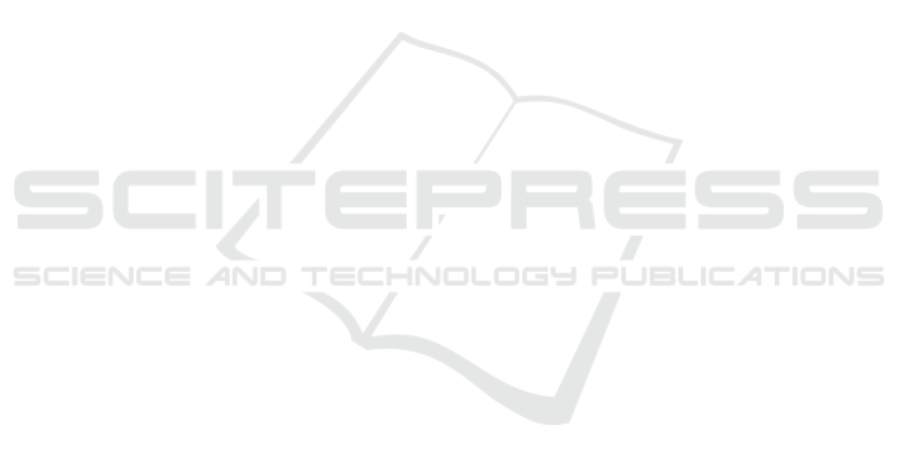
recursive functions, e.g., the Ackermann function of
(3, 7) was computed 3.65 times faster; the 30th Fi-
bonacci number was computed 3.31 times faster. The
speedup factor of computation-intensive loops ranges
from 1.24 to 2.64. Although such significant improve-
ments could not be achieved in real-world applica-
tions, validation on a WordPress website has shown
the safety of our optimizations, as they never worsen
the performance nor change the program semantics.
In our future work, we plan to extend the special-
ization mechanism even further and improve its ef-
fect on real-life websites, e.g., by producing clones
not only of global functions but of class methods as
well. Moreover, procedure inlining will be explored
as another possible context-sensitive interprocedural
optimization in dynamic language AOT compilers.
ACKNOWLEDGEMENTS
This work has been supported by the Czech Science
Foundation project no. 20-07487S, Charles Univer-
sity Grant Agency (GA UK) project no. 896120, the
project PROGRESS Q48 and the grant SVV-260588.
REFERENCES
Abonyi, A., Balas, D., Be
ˇ
no, M., M
´
ı
ˇ
sek, J., and Zavoral,
F. (2009). Phalanger improvements. Technical report,
Charles University in Prague.
Aho, A. V., Lam, M. S., Sethi, R., and Ullman, J. D. (2006).
Compilers: Principles, Techniques, and Tools (2nd
Edition). Addison-Wesley Longman.
Alm
´
asi, G. and Padua, D. (2002). MaJIC: Compiling MAT-
LAB for speed and responsiveness. SIGPLAN Not.,
37(5):294–303.
Benda, J., Matousek, T., and Prosek, L. (2006). Phalanger:
Compiling and running PHP applications on the Mi-
crosoft .NET platform. .NET Technologies 2006.
Biggar, P. (2010). Design and Implementation of an Ahead-
of-Time Compiler for PHP. PhD thesis, Trinity Col-
lege Dublin.
Biggar, P., de Vries, E., and Gregg, D. (2009). A practical
solution for scripting language compilers. In SAC ’09,
page 1916–1923. ACM.
Chambers, C. and Ungar, D. (1991). Making pure object-
oriented languages practical. In OOPSLA ’91, page
1–15. ACM.
Chevalier-Boisvert, M. and Feeley, M. (2014). Simple and
effective type check removal through lazy basic block
versioning. CoRR, abs/1411.0352.
Chevalier-Boisvert, M., Hendren, L., and Verbrugge, C.
(2010). Optimizing matlab through just-in-time spe-
cialization. In CC ’10, page 46–65. Springer-Verlag.
Cooper, K. D., Hall, M. W., and Kennedy, K. (1993). A
methodology for procedure cloning. Computer Lan-
guages, 19(2):105–117. ICCL ’92.
Damas, L. and Milner, R. (1982). Principal type-schemes
for functional programs. In POPL ’82, page 207–212.
ACM.
Dean, J., Chambers, C., and Grove, D. (1995). Selec-
tive specialization for object-oriented languages. SIG-
PLAN Not., 30(6):93–102.
Emami, M., Ghiya, R., and Hendren, L. J. (1994).
Context-sensitive interprocedural points-to analysis in
the presence of function pointers. SIGPLAN Not.,
29(6):242–256.
Hus
´
ak, R., Zavoral, F., and Kofro
ˇ
n, J. (2020). Optimiz-
ing transformations of dynamic languages compiled
to intermediate representations. In TASE ’20, pages
145–152.
M
´
ı
ˇ
sek, J., Fistein, B., and Zavoral, F. (2016). Inferring com-
mon language infrastructure metadata for an ambigu-
ous dynamic language type. In ICOS ’16, pages 111–
116.
M
´
ı
ˇ
sek, J. and Zavoral, F. (2010). Mapping of dynamic lan-
guage constructs into static abstract syntax trees. In
ICIS ’10, pages 625–630.
M
´
ı
ˇ
sek, J. and Zavoral, F. (2017). Control flow ambiguous-
type inter-procedural semantic analysis for dynamic
language compilation. Procedia Computer Science,
109:955–962. ANT ’17 and SEIT ’17.
M
´
ı
ˇ
sek, J. and Zavoral, F. (2019). Semantic analysis of
ambiguous types in dynamic languages. Journal
of Ambient Intelligence and Humanized Computing,
10(7):2537–2544.
Ottoni, G. (2018). HHVM JIT: A profile-guided, region-
based compiler for PHP and Hack. In PLDI ’18, page
151–165. ACM.
Rigo, A. and Pedroni, S. (2006). PyPy’s approach to vir-
tual machine construction. In OOPSLA ’06, page
944–953. ACM.
Serrano, M. (2018). Javascript AOT compilation. In DLS
’18, DLS 2018, page 50–63. ACM.
Tobin-Hochstadt, S. and Felleisen, M. (2010). Logi-
cal types for untyped languages. SIGPLAN Not.,
45(9):117–128.
Wimmer, C. and W
¨
urthinger, T. (2012). Truffle: A self-
optimizing runtime system. In SPLASH ’12, page
13–14. ACM.
Zhao, H., Proctor, I., Yang, M., Qi, X., Williams, M., Gao,
Q., Ottoni, G., Paroski, A., MacVicar, S., Evans, J.,
and Tu, S. (2012). The hiphop compiler for php. SIG-
PLAN Not., 47(10):575–586.
ICSOFT 2022 - 17th International Conference on Software Technologies
186