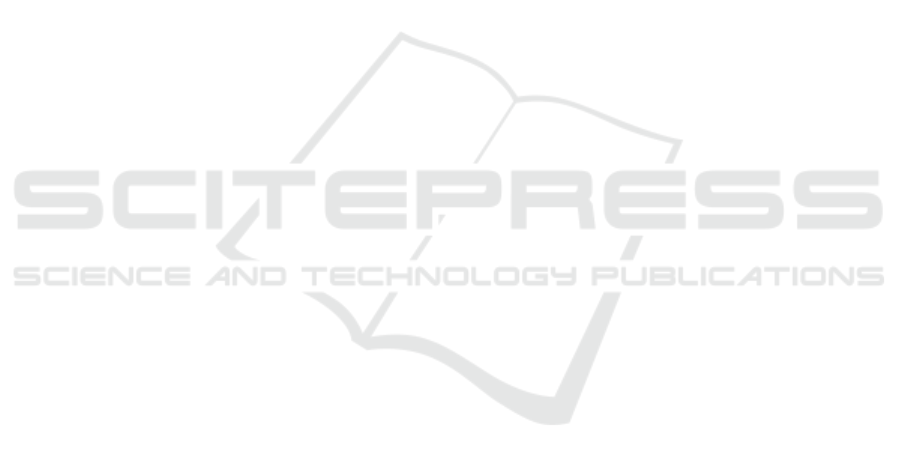
Cockburn, A. (2005). Hexagonal architecture. http://alistair
.cockburn.us/Hexagonal+architecture. Last accessed:
08/22/18.
Coplien, J. and Bjørnvig, G. (2011). Lean Architecture: for
Agile Software Development. Wiley.
Czaplicki, E. (2016). The elm architecture. https://guide.el
m-lang.org/architecture/. Last accessed: 04/29/22.
Dobrean, D. and Dios
,
an, L. (2019). A comparative study of
software architectures in mobile applications. Studia
Universitatis Babes
,
-Bolyai Informatica, 64(2):49–64.
Evans, E. (2003). Domain-Driven Design. Addison-Wesley
Professional, Boston, MA.
Gamma, E., Helm, R., Johnson, R., and Vlissides, J.
(1995). Design Patterns. Elements of Reusable
Object-Oriented Software. Addison-Wesley, Boston,
MA.
Gilbert, J. and Stoll, C. (2014). Architecting ios apps with
viper. https://www.objc.io/issues/13-architecture/vip
er/. Last accessed: 04/29/22.
Graça, H. (2017). Ddd, hexagonal, onion, clean, cqrs, . . .
how i put it all together. https://herbertograca.com/
2017/11/16/explicit-architecture-01-ddd-hexagona
l-onion-clean-cqrs-how-i-put-it-all-together/. Last
accessed: 04/29/22.
Jacobson, I., Christerson, M., Jonsson, P., and Övergaard,
G. (1992). Object-Oriented Software Engineering.
ACM, New York, NY, USA.
Knott, D. (2015). Hands-On Mobile App Testing. Addison-
Wesley Professional, Boston, MA.
König-Ries, B. (2009). Challenges in mobile application
development. it Inf. Technol., 51(2):69–71.
Kušt, I. (2019). Clean architecture tutorial for android: Get-
ting started. https://www.raywenderlich.com/359591
6-clean-architecture-tutorial-for-android-getting-star
ted. Last accessed: 04/29/22.
La, H. J. and Kim, S. D. (2010). Balanced mvc archi-
tecture for developing service-based mobile applica-
tions. In 2010 IEEE 7th International Conference on
E-Business Engineering, pages 292–299.
Law, R. (2019). The Clean Swift Handbook.
Martin, R. and Martin, M. (2006). Agile Principles, Pat-
terns, and Practices in C#. Robert C. Martin Series.
Pearson Education.
Martin, R. C. (2017). Clean Architecture - A Craftsman’s
Guide to Software Structure and Design. Prentice
Hall, Englewood Cliffs, NJ.
Nunkesser, R. (2018). Beyond web/native/hybrid: A new
taxonomy for mobile app development. In Proceed-
ings of the 5th International Conference on Mobile
Software Engineering and Systems, MOBILESoft ’18,
Piscataway, NJ. IEEE Press.
Palermo, J. (2008). Onion architecture. https://jeffreypa
lermo.com/2008/07/the-onion-architecture-part-1/.
Last accessed: 04/29/22.
Plakalovic, D. and Simic, D. (2010). Applying mvc
and pac patterns in mobile applications. ArXiv,
abs/1001.3489.
Rafi, D. M., Moses, K. R. K., Petersen, K., and Mäntylä,
M. V. (2012). Benefits and limitations of automated
software testing: Systematic literature review and
practitioner survey. In 2012 7th International Work-
shop on Automation of Software Test (AST), pages 36–
42.
Reenskaug, T. (1979). Models - views - controllers. Tech-
nical report, Xerox PARC.
Salazar, F. J. A. and Brambilla, M. (2015). Tailoring soft-
ware architecture concepts and process for mobile ap-
plication development. In Proceedings of the 3rd In-
ternational Workshop on Software Development Life-
cycle for Mobile, DeMobile 2015, page 21–24, New
York, NY, USA. Association for Computing Machin-
ery.
Shahbudin, F. E. and Chua, F. (2013). Design patterns for
developing high efficiency mobile application. Jour-
nal of Information Technology & Software Engineer-
ing, 3:1–9.
Sokolova, K. and Lemercier, M. (2014). Towards high qual-
ity mobile applications: Android passive mvc archi-
tecture. International Journal On Advances in Soft-
ware 1942-2628, 7:123 – 138.
Sommerville, I. (2016). Software Engineering. Pearson,
London, 10th edition.
Sommerville, I. (2020). Engineering Software Products.
Pearson, London.
Staltz, A. (2015). Model-view-intent. https://cycle.js.org/m
odel-view-intent.html. Last accessed: 04/29/22.
Vernon, V. (2013). Implementing Domain-Driven Design.
Addison-Wesley Professional.
Vollmer, G. (2017). Mobile App Engineering: Von den Re-
quirements zum Go Live. dpunkt.verlag, Heidelberg.
Wasserman, A. (2010). Software engineering issues for mo-
bile application development. pages 397–400.
Wichmann, D., Pielot, M., and Boll, S. (2009). Companion
platform - modulare softwareplattform zur schnellen
entwicklung von mobilen anwendungen. it - Informa-
tion Technology, 51(2):72 – 78.
ICSOFT 2022 - 17th International Conference on Software Technologies
120