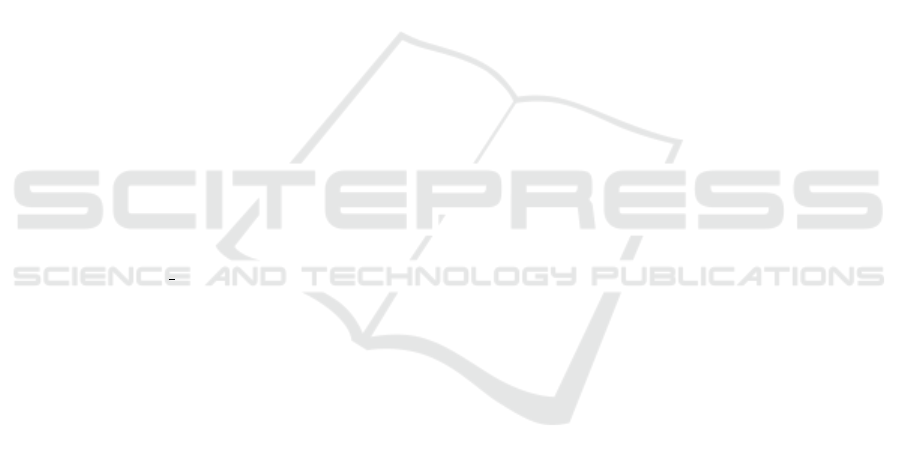
In the second approach, the compiler transforms
the program to translate coroutines into an equivalent
program without coroutines. This is the approach fol-
lowed by most generators such as in C#, JavaScript,
and others.
We claim that leash to co-routines induce heavy-
weight approaches that incur in performance over-
heads on generators. On the other hand, object-
oriented iterators incur in useless complexity and ver-
bosity that affects readability and expressiveness of
streams operations (Baker, 1993). The tinyield design
proposal suppresses those limitations with advantages
in both performance and extensibility conciseness.
Our model has been already extended for asyn-
chronous processing and has evidences that may over-
take alternatives such as reactive streams (Kaazing
et al., 2017) achieving better throughput under some
non-blocking IO scenarios. Previous work has al-
ready been made in this field (Prokopec and Liu,
2018) but again, it is tight with co-routines subject,
which we have shown in this work that is harmful for
streams traversal, namely in JavaScript environment.
ACKNOWLEDGEMENTS
This work was supported by Instituto Politec-
nico de Lisboa, Lisbon, Portugal, for funding
the projet ”Reactive Web streams for Big Data”
(IPL/2020/WebFluid ISEL).
REFERENCES
(2020). ECMAScript 2020 language specification, 11th edi-
tion. ECMA, 11 edition.
Baker, H. G. (1993). Iterators: Signs of weakness in object-
oriented languages. SIGPLAN OOPS Mess., 4(3):18–
25.
Borins, M., Braun, A. R., Palmer, R., and Terlson,
B. (2006). ECMA-334 C# language specification.
ECMA, 5 edition.
Bynens, M. and Dalton, J.-D. (2014). benchmarkjs: A
benchmarking library that supports high-resolution
timer.
Carvalho, F. M., Duarte, L., and Gouesse, J. (2020). Text
web templates considered harmful. In Web Informa-
tion Systems and Technologies, pages 69–95, Cham.
Springer International Publishing.
Conway, M. E. (1963). Design of a separable transition-
diagram compiler. Commun. ACM, 6(7):396–408.
CTS (2012). ECMA-335 Common Language Infrastructure
(CLI), 6th edition, June 2012. ECMA, 6 edition.
Dragos, I., Cunei, A., and Vitek, J. (2007). The-
ory and practice of coroutines with snapshots. In
ICOOOLPS’2007, Technische Universit
¨
at Berlin.
Fowler, M. (2015). Collection pipeline.
Friedman, D. P. and Wise, D. S. (1976). CONS should not
evaluate its arguments. In Michaelson, S. and Milner,
R., editors, Automata, Languages and Programming,
pages 257–284, Edinburgh, Scotland. Edinburgh Uni-
versity Press.
Hunt, A. and Thomas, D. (2003). The art of enbugging.
IEEE SOFTWARE.
James, R. and Sabry, A. (2011). Yield: Mainstream de-
limited continuations. Workshop on the Theory and
Practice of Delimited Continuations.
Jones, S. (2003). Haskell 98 Language and Libraries: The
Revised Report. Journal of functional programming:
Special issue. Cambridge University Press.
Kaazing, Lightbend, Netflix, Pivotal, and Hat, R. (2017).
Reactive streams specification for the jvm.
Landin, P. J. (1964). The Mechanical Evaluation of Expres-
sions. The Computer Journal, 6(4):308–320.
Landin, P. J. (1965). Correspondence between algol 60
and church’s lambda-notation: Part i. Commun. ACM,
8(2):89–101.
Langer, A. and Kreft, K. (2015). Stream performance. JAX
London Online Conference.
Liskov, B. (1983). CLU Reference Manual. Springer-Verlag
New York, Inc., Secaucus, NJ, USA.
Liskov, B. (1996). A History of CLU, page 471–510. Asso-
ciation for Computing Machinery, NY, USA.
OpenJDK (2020). Loom - fibers, continuations and tail-
calls for the jvm.
Parlog, N. (2019). Github.
Poeira, D. and Carvalho, F. M. (2020). Benchmark for dif-
ferent sequence operations in java and kotlin. Tech-
nical report, https://github.com/tinyield/sequences-
benchmarks.
Prokopec, A. and Liu, F. (2018). Theory and practice of
coroutines with snapshots. In European Conference
on Object-Oriented Programming.
Ryzhenkov, I. (2014). JetBrains.
Shaw, M., Wulf, W. A., and London, R. L. (1977). Ab-
straction and verification in alphard: Defining and
specifying iteration and generators. Commun. ACM,
20(8):553–564.
Shipilev, A. (2013). Java microbenchmark harness (the
lesser of two evils).
Stadler, L., Wimmer, C., W
¨
urthinger, T., M
¨
ossenb
¨
ock, H.,
and Rose, J. (2009). Lazy continuations for java vir-
tual machines. In Proceedings of the 7th International
Conference on Principles and Practice of Program-
ming in Java, PPPJ ’09, page 143–152, New York,
NY, USA. Association for Computing Machinery.
Thomas, D. and Hunt, A. (2007). Programming Ruby: The
Pragmatic Programmer’s Guide. Addison-Wesley.
Yee, K.-P. and van Rossum, G. (2001). Pep 234 – iterators.
Technical report, Python.
ICSOFT 2021 - 16th International Conference on Software Technologies
150