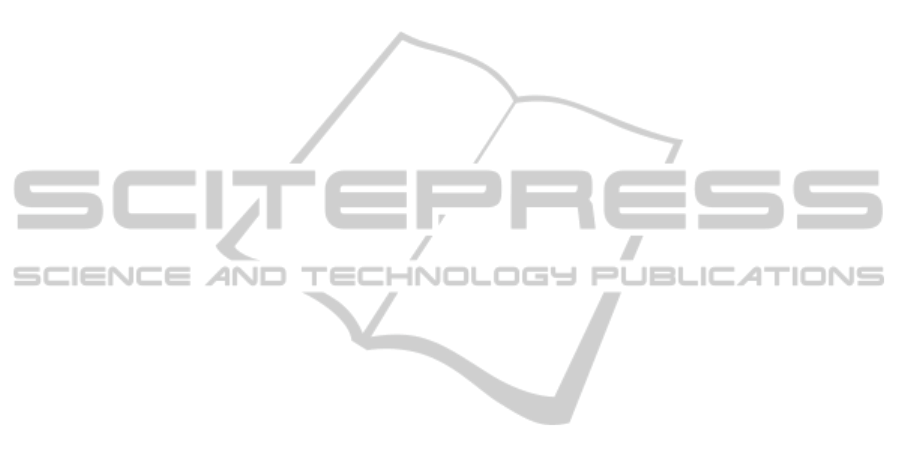
specifically those supporting C++11’s thread and
chrono libraries (Josuttis, 2012). We argue that this
library’s timers offer an easier and more advanced
API comparatively to two other important timer
libraries. Besides functional advantages, the
proposed library also surpasses the others in terms of
temporization performance.
In the following section we review related work.
In section III, two widely used libraries are analysed
from the perspective of a varied set of usage
scenarios. At the same time, we present how those
scenarios can be supported by an easier to use API.
Section IV describes the proposed library and
performance measurements. Finally, we present
conclusions and future work.
2 RELATED WORK
We are interested in a timer that waits a given
interval of time without blocking the program, and if
it is not stopped before that interval expires (i.e.,
timeout event occurs) it calls a pre-configured
function. We refer to such a function as callback,
and it is executed asynchronously to the rest of the
program. Therefore, it is an event-handler as found
in event-driven programming environments.
In our search we were able to find several C++
libraries that include timers. As mentioned in the
previous section, all of them depende on platform
specific code, i.e., they are not based on C++11
standard library.
In the low-level library (Mitchell, 2013), the
developer is responsible for implementing the events
loop. The specific event-driven design of (The Qt
Company 2015) applies to timers, whose events are
dispatched as all others, and timers can only be
controlled from the thread that creates them. In
(Robinson, 2013), timer callbacks are executed by
the thread that dispatches events, thus blocking other
(timer) events. These event processing limitations
are not found in (Henning, 2004; King, 2009). In
(Henning 2004), timers are implemented by deriving
a base class and a thread is launched for each timer
object. In (King 2009) there is a thread dedicated to
process all timers, which launches one thread to
execute each callback.
Two other libraries are reviewed in more detail
since they are used in the following section. Boost is
a large and important collection of C++ libraries that
has had a major influence on C++ standard
evolution. It includes Asio (Kohlhoff, 2014), a
library that supports timers. When an Asio timer is
created an io_service is associated to it. One or more
callbacks are attached to a timer by using its
async_wait function. The time interval starts at timer
creation, and the io_service::run function blocks if
invoked before the interval end, otherwise it
executes the callbacks. Consequently, Asio timers
requires the addition of multithreading to obtain
asynchronous temporization as defined in the
previous section. It is a lower-level library.
Poco (Applied Informatics Software Engineering
2010) is also a collection of libraries that offers two
kinds of timers. One is the Poco::Util::Timer, which
similarly to a Java timer works as a timed task
scheduler, executing callbacks in sequence. The
other one is Poco::Timer, from the main library, that
is closer to the one proposed in this paper, whose
model is described in the previous section. Although
the former can be used according to that model, we
hereafter consider the latter. The timer is created by
specifying a start interval and a periodic interval.
Callback objects are created from user classes using
the TimerCallback template, which allows using any
user function that has the first parameter of type
Poco::Timer. The callback object is then passed to
the timer using its start function, which begins the
temporization. If the timer is not stopped before
expiry, the callback is executed by an internal pool
of threads.
3 TIMER USE CASES
This section discusses the implementation of
solutions to temporization scenarios using Asio and
Poco. At the same time, our view of an easier to use
API is anticipated.
3.1 Single Temporization
In this scenario the timer is started once to execute a
given callback when it expires.
In consequence of the general description of
Asio timers previously given, it is necessary to
create a thread to avoid blocking the main thread.
Asio callbacks must be void and accept as first
parameter an error code. The code in figure 1
considers a callback that also receives an integer
number, for example purposes. Consequently,
async_wait needs a binding of the extra arguments.
After expiration and callback execution, the
io_sevice::run function returns and ends the thread.
The Poco implementation is given in figure 2, in
which timer arguments specify, respectively, the
start and periodic intervals. The callback function
must be void and have only one parameter of the