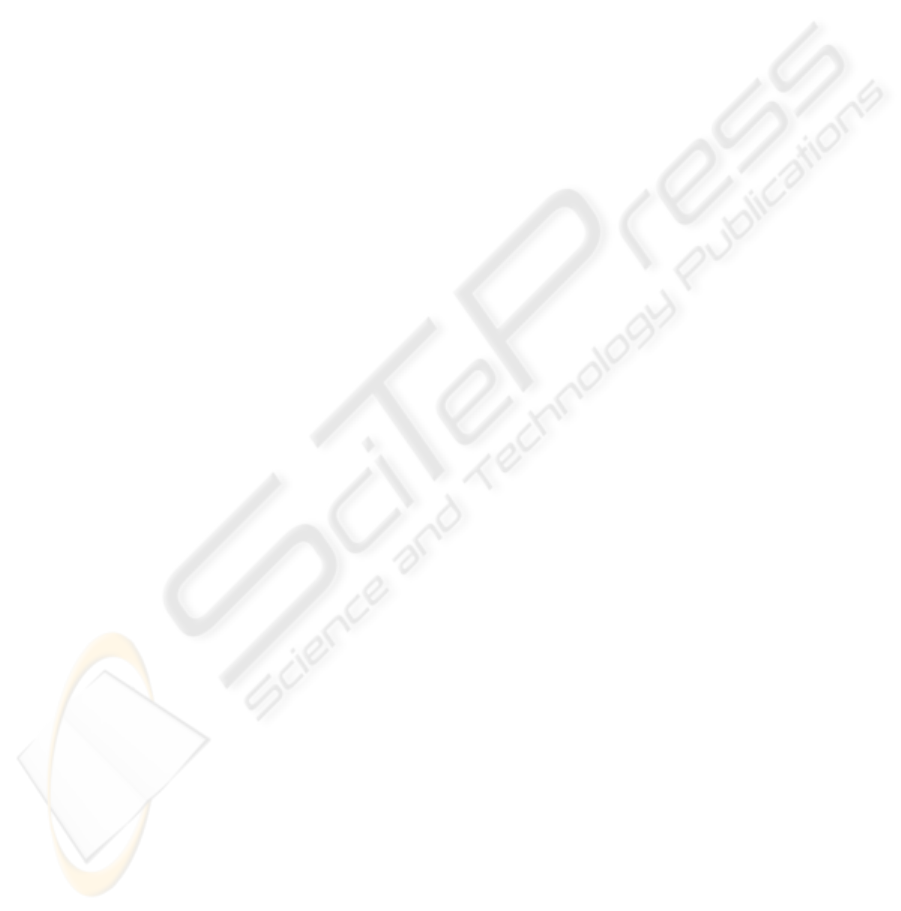
mance increases rapidly even with a small ray packet.
This is due to the higher probability of finding coher-
ent rays. In contrast, non coherent rays show a slight
ascending trend as the packet size increases. A larger
packet size implies higher probabilities of being able
to parallelize the calculations; the maximum possi-
ble efficiency is obtained with our algorithm since the
packet contains all the available rays.
4 CONCLUSIONS
Until now, acceleration techniques for ray-tracing
were useful only when it was easy to find coherent
rays to process in parallel. This article introduces a
ray tracing algorithm which instead of traversing the
spatial indexing of the scene once per ray or per ray
packet, it traverses the tree only once with all rays si-
multaneously (or a maximum of eight times if the rays
have director vectors of different sign).
This technique has been shown to present the fol-
lowing advantages: It can extract a higher level of
parallelism in ray processing, even for non coherent
rays, and a node will be visited at most eight times,
resulting in minimization of memory accesses.
Future work includes disabling the ray stack
scheme when the number of rays in a node is small,
to increase efficiency, studying wider SIMD arquitec-
tures, since speedup should be higher (Benthin et al.,
2006), out of core extensions and implementation on
GPUs. A battery of tests with large complete scenes
will validate applicability in real-world scenarios.
ACKNOWLEDGEMENTS
This article has been partially financed by projects
TIN2004-07672-C03-02 of the Spanish Ministry
of Education and Science, and P06-TIC-01403 of
the Junta de Andaluc
´
ıa and the European Union
(FEDER).
REFERENCES
Appel, A. (1968). Some techniques for shading machine
renderings of solids. In AFIPS 1968 Spring Joint
Computer Conf., volume 32, pages 37–45.
Benthin, C., Wald, I., Scherbaum, M., and Friedrich, H.
(2006). Ray tracing on the cell processor. rt, 0:15–
23.
Boulos, S., Wald, I., and Benthin, C. (2008). Adaptive ray
packet reordering. Interactive Ray Tracing, 2008. RT
2008. IEEE Symposium on, pages 131–138.
Carr, N. A., Hall, J. D., and Hart, J. C. (2002). The ray
engine. In HWWS ’02, pages 37–46, Aire-la-Ville,
Switzerland. Eurographics Association.
Christen, M. (2005). Ray tracing on gpu. Master’s thesis,
University of Applied Sciences Basel, Switzerland.
Foley, T. and Sugerman, J. (2005). Kd-tree acceleration
structures for a gpu raytracer. In HWWS ’05, pages
15–22, New York, NY, USA. ACM.
Gribble, C. P. and Ramani, K. (2008). Coherent ray tracing
via stream filtering. Interactive Ray Tracing, 2008. RT
2008. IEEE Symposium on, pages 59–66.
Hanrahan, P. (1986). Using caching and breadth-first search
to speed up ray-tracing. In Proceedings on Graphics
Interface ’86/Vision Interface ’86, pages 56–61.
Intel Corporation (2008). Intel 64 and IA-32 Architectures
Software Developer’s Manual Volume 1: Basic Archi-
tecture.
Jensen, H. W. (1996). Global Illumination Using Photon
Maps. In Rendering Techniques ’96, pages 21–30.
Mahovsky, J. and Wyvill, B. (2006). Memory-conserving
bounding volume hierarchies with coherent raytrac-
ing. Comput. Graph. Forum, 25(2):173–182.
M
¨
oller, T. and Trumbore, B. (1997). Fast, minimum storage
ray-triangle intersection. journal of graphics tools,
2(1):21–28.
Noguera, J. M. and Ure
˜
na, C. (2007). Un algoritmo de
recorrido vectorizado para ray-tracing. In CEDI-
CEIG’07: Proceedings of the XVII Congreso Espa
˜
nol
de Inform
´
atica Gr
´
afica, pages 41–50.
Pharr, M., Kolb, C., Gershbein, R., and Hanrahan, P. (1997).
Rendering complex scenes with memory-coherent ray
tracing. In SIGGRAPH ’97, pages 101–108.
Purcell, T. J., Buck, I., Mark, W. R., and Hanrahan, P.
(2002). Ray tracing on programmable graphics hard-
ware. ACM Transactions on Graphics, 21(3):703–
712.
Revelles, J., Ure
˜
na, C., and Lastra, M. (2000). An ef-
ficient parametric algorithm for octree traversal. In
WSCG’2000, pages 212–219.
Santalo, L. A. (1976). Integral geometry and geometric
probability. Addison-Wesley, Massachusetts (etc.).
Sbert, M. (1993). An integral geometry based method for
fast form-factor computation. Computer Graphics Fo-
rum (Eurographics ’93), 12(3):409–420.
Wald, I. (2004). Realtime Ray Tracing and Interactive
Global Illumination. PhD thesis, Saarland University.
Wald, I., Benthin, C., Wagner, M., and Slusallek, P. (2001).
Interactive rendering with coherent ray tracing. In
Computer Graphics Forum, volume 20(3), pages 153–
164.
Wald, I., Gribble, C. P., Boulos, S., and Kensler, A. (2007).
SIMD Ray Stream Tracing. In Symposium on Interac-
tive Ray Tracing, Conference Program, page 24.
A VECTORIZED TRAVERSAL ALGORITHM FOR RAY TRACING
63