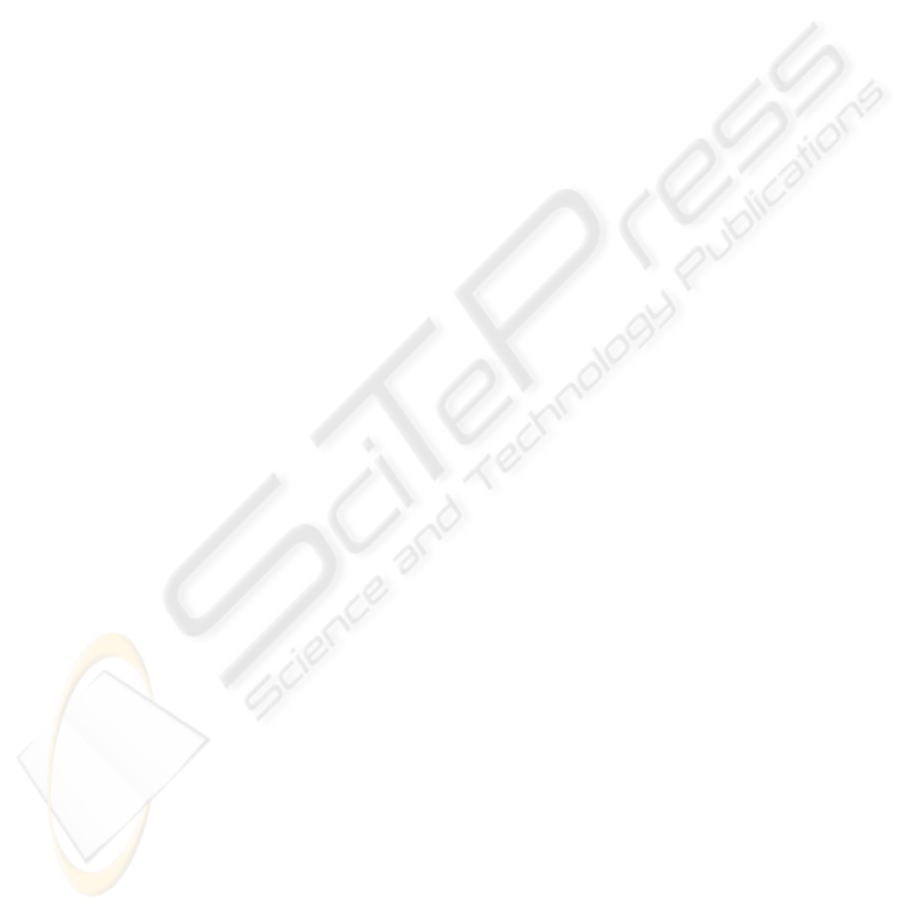
ACKNOWLEDGEMENTS
The research described in this paper received support
from the Natural Sciences and Engineering Research
Council of Canada. We thank the reviewers for their
helpful comments.
REFERENCES
Ada (1995). Ada 95 Reference Manual. Re-
vised International Standard ISO/IEC 8652:1995.
www.adahome.com/rm95. Accessed 2008/03/12.
Armstrong, J. (2007). A history of Erlang. In HOPL
III: Proceedings of the Third ACM SIGPLAN Confer-
ence on the History of Programming Languages, New
York, NY, USA, pp. 6.1–6.26. ACM Press.
Barnes, F. R. and P. H. Welch (2006). Occam-π :
blending the best of CSP and the π-calculus.
www.cs.kent.ac.uk/projects/ofa/kroc. Accessed
2008/03/13.
Benton, N., L. Cardelli, and C.Fournet (2004, Septem-
ber). Modern concurrency abstractions for C]. ACM
Transactions on Programming Languages and Sys-
tems 26(5), 769–804.
Boehm, H.-J. (2005). Threads cannot be implemented as a
library. In Proceedings of the 2005 ACM SIGPLAN
Conference on Programming Language Design and
Implementation (PLDI ’05), pp. 261–268.
Brinch Hansen, P. (1987, January). Joyce—a programming
language for distributed systems. Software—Practice
and Experience 17(1), 29–50.
Brinch Hansen, P. (1996). The Search for Simplicity—
Essays in Parallel Programming. IEEE Computer So-
ciety Press.
Dijkstra, E. W. (1968). Cooperating sequential processes. In
F. Genuys (Ed.), Programming Languages: NATO Ad-
vanced Study Institute, pp. 43–112. Academic Press.
Grogono, P. and B. Shearing (2008). MEC Reference
Manual. http://users.encs.concordia.ca/∼grogono/-
Erasmus/erasmus.html.
G
¨
untensperger, R. and J. Gutknecht (2004, May). Active
C]. In 2nd International Workshop .NET Technolo-
gies’2004, pp. 47–59.
Henzinger, T. A., R. Jhala, and R. Majumdar (2005). Per-
missive interfaces. In ESEC/FSE-13: Proceedings of
the 10th European Software Engineering Conference
(held jointly with 13th ACM SIGSOFT International
Symposium on Foundations of Software Engineering),
pp. 31–40.
Hoare, C. A. R. (1974). Hints on programming language
design. In C. Bunyan (Ed.), Computer Systems Relia-
bility, Volume 20 of State of the Art Report, pp. 505–
534. Pergamon. Reprinted in (Hoare and Jones, 1989).
Hoare, C. A. R. (1978, August). Communicating sequential
processes. Communications of the ACM 21(8), 666–
677.
Hoare, C. A. R. and C. Jones (1989). Essays in Computing
Science. Prentice Hall.
Hunt, G. C. and J. R. Larus (2007). Singularity: rethinking
the software stack. SIGOPS Operating System Review
41(2), 37–49.
Jackson, M. (1978). Information systems: Modelling, se-
quencing and transformation. In Proceedings of the
3rd International Conference on Software Engineer-
ing (ICSE 1978), pp. 72–81.
Jackson, M. (1980). Information systems: Modelling, se-
quencing and transformation. In R. McKeag and A.
MacNaughten (Eds.), On the Construction of Pro-
grams. Cambridge University Press.
Kay, A. C. (1993). The early history of Smalltalk. ACM
SIGPLAN Notices 28(3), 69–95.
Khorfage, W. and A. P. Goldberg (1995, April). Hermes
language experiences. Software—Practice and Expe-
rience 25(4), 389–402.
Lameed, N. and P. Grogono (2008). Separating program
semantics from deployment. These proceedings.
Lea, D., P. Soper, and M. Sabin (2004). The Java Iso-
lation API: Introduction, applications and inspira-
tion. bitser.net/isolate-interest/slides.pdf. Accessed
2007/06/14.
Lee, E. A. (2006, May). The problem with threads. IEEE
Computer 39(5), 33–42.
Matsuoka, S. and A. Yonezawa (1993). Analysis of in-
heritance anomaly in object-oriented concurrent pro-
gramming language. In Research Directions in Con-
current Object-Oriented Programming, pp. 107–150.
MIT Press.
May, D. (1983, April). Occam. ACM SIGPLAN No-
tices 18(4), 69–79.
Milicia, G. and V. Sassone (2004). The inheritance
anomaly: ten years after. In SAC ’04: Proceedings
of the 2004 ACM Symposium on Applied Computing,
New York, NY, USA, pp. 1267–1274. ACM.
Milner, R. (1980). A Calculus of Communicating Systems.
Springer.
Milner, R. (1999). Communicating and Mobile Systems:
The π Calculus. Cambridge University Press.
Nygaard, K. and O.-J. Dahl (1981). The development of the
SIMULA language. In R. Wexelblat (Ed.), History
of Programming Languages, pp. 439–493. Academic
Press.
Object Management Group (2007, November). OMG Uni-
fied Modeling Language (OMG UML), Superstruc-
ture, V2.1.2. http://www.omg.org/spec/UML/2.1.2/-
Superstructure/PDF/. Accessed 2008/03/15.
Soper, P. (2002). JSR 121: Application Isola-
tion API Specification. Java Specification Re-
quests. http://jcp.org/aboutJava/communityprocess/-
final/jsr121/index.html. Accessed 2008/03/15.
Strom, R. (1991). HERMES: A Language for Distributed
Computing. Prentice Hall.
Sutter, H. and J. Larus (2005, September). Software and the
concurrency revolution. ACM Queue 3(7), 54–62.
van Roy, P. and S. Haridi (2001). Concepts, Techniques,
and Models of Computer Programming. MIT Press.
ICSOFT 2008 - International Conference on Software and Data Technologies
54