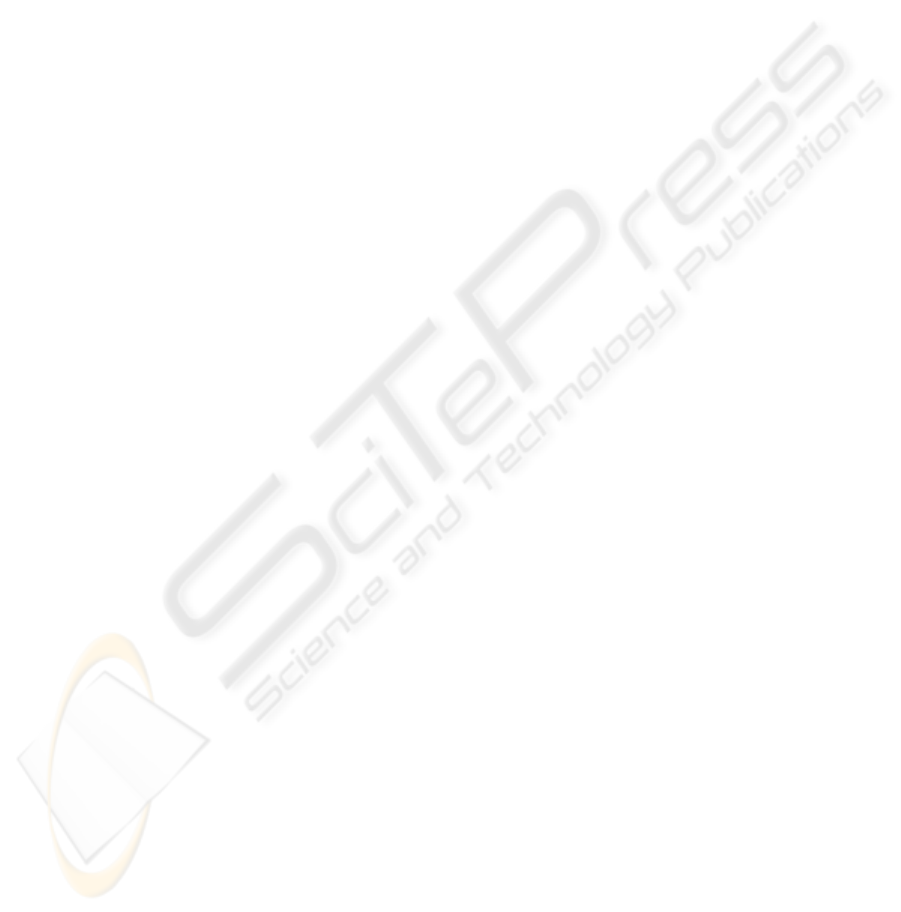
rializations. Our solution allows to implement gener-
ically the serialization and the optimization described
in Sect. 2, without imposing run-time overhead. Sim-
ilarly, any type-dependent operation of the specified
kind can be implemented.
We extract field information from class defini-
tions and represent it in containers that model stati-
cally indexed families. It is known how to implement
such containers, but shortcomings of conventional im-
plementations have to be resolved for our purpose.
In particular, support of unusual element types and
proper initialization need to be ensured. Linear al-
gorithms are expressed elegantly in terms of higher-
order combinators. Optimizations and other features
are beneficial for either use of the construct as generic
container or as internal class representation.
Class definitions that inherit their fields from a
container involve boilerplate code. We propose to
share that code in a wrapper that makes the container
a record. We accept a conflict with the principle of
encapsulation and leave a more complete solution to
future work on component-based class composition.
As it relies on static meta-programming, our so-
lution pays run-time efficiency by increased compi-
lation efforts. We consider these costs tolerable for
performance-critical systems, and for objects with
rather few fields. In other cases, dynamic reflection
may be more appropriate.
Our approach attempts to deal with as many as-
pects and uses of field definition and reflection as pos-
sible within the underlying container. But we do not
claim or require their complete coverage. A class def-
inition in the proposed style allows to influence or
override any behavior of the underlying family. Only
essential and invariable properties are encapsulated.
The solution is a flexibly applicable implementa-
tion pattern, sufficiently abstract to be expressed en-
tirely in terms of portable library components, and
that does not rely on compiler extensions. We can
conveniently add extensions to the components, that
automatically enrich the semantics of all classes de-
fined by that pattern. We consider these aspects sig-
nificant advantages of our solution over alternative
approaches that rely on language extensions and com-
piler facilities. These solutions are safer to use, but
harder to propagate and extend.
ACKNOWLEDGEMENTS
We are grateful to Gustav Munkby and Marcin Za-
lewski for inspiring discussions, to the reviewers for
useful comments, and to the Swedish Research Coun-
cil (Vetenskapsr
˚
adet) for supporting this work in part.
REFERENCES
Attardi, G. and Cisternino, A. (2001). Reflection Support
by Means of Template Metaprogramming. In Proc.
3rd Int. Conf. on Generative and Component-Based
Software Engineering (GCSE), volume 2186 of LNCS,
pages 118–127. Springer.
Bracha, G. and Cook, W. (1990). Mixin-Based Inheritance.
In Proc. 5th ACM Symp. on Object Oriented Program-
ming: Systems, Languages and Applications (OOP-
SLA), volume 25, number 10 of ACM SIGPLAN No-
tices, pages 303–311. ACM Press.
Calcagno, C., Taha, W., Huang, L., and Leroy, X. (2003).
Implementing Multi-Stage Languages using ASTs,
Gensym, and Reflection. In Proc. 2nd Int. Conf. on
Generative Programming and Component Engineer-
ing (GPCE), volume 2830 of LNCS, pages 57–76.
Springer.
Czarnecki, K. and Eisenecker, U. W. (2000). Genera-
tive Programming: Methods, Tools and Applications.
Addison-Wesley.
de Guzman, J. and Marsden, D. (2006). Fusion Li-
brary Homepage.
http://spirit.sourceforge.
net/dl_more/fusion_v2/libs/fusion
.
Eisenecker, U. W., Blinn, F., and Czarnecki, K. (2000). A
Solution to the Constructor-Problem of Mixin-Based
Programming in C++. In Proc. First Workshop on
C++ Template Programming at 2nd GCSE.
Hinze, R. and L
¨
oh, A. (2006). “Scrap Your Boilerplate”
Revolutions. In Uustalu, T., editor, Proc. 8th Int. Conf.
on Mathematics of Program Construction (MPC), vol-
ume 4014 of LNCS, pages 180–208. Springer.
J
¨
arvi, J. (1999). Tuples and Multiple Return Values in
C++. Technical Report 249, Turku Centre for Com-
puter Science.
J
¨
arvi, J. (2001). Boost Tuple Library Homepage.
http:
//www.boost.org/libs/tuple
.
L
¨
ammel, R. and Peyton Jones, S. (2003). Scrap Your Boil-
erplate: A Practical Design Pattern for Generic Pro-
gramming. In Proc. ACM SIGPLAN Int. Workshop
on Types in Language Design and Implementation
(TLDI), volume 38, number 3 of ACM SIGPLAN No-
tices, pages 26–37. ACM Press.
Munkby, G., Priesnitz, A., Schupp, S., and Zalewski, M.
(2006). Scrap++: Scrap Your Boilerplate in C++.
In Proc. 2006 ACM SIGPLAN Workshop on Generic
Programming (WGP), pages 66–75. ACM Press.
Smaragdakis, Y. and Batory, D. (2000). Mixin-Based Pro-
gramming in C++. In Proc. 2nd Int. Symp. on Gen-
erative and Component-Based Software Engineer-
ing (GCSE), volume 2177 of LNCS, pages 163–177.
Springer.
Weiss, R. and Simonis, V. (2003). Storing properties in
grouped tagged tuples. In Proc. 5th Int. Conf. on Per-
spectives of Systems Informatics (PSI), volume 2890
of LNCS, pages 22–29. Springer.
Winch, E. (2001). Heterogeneous Lists of Named Ob-
jects. In Proc. Second Workshop on C++ Template
Programming at 16th OOPSLA.
A PATTERN FOR STATIC REFLECTION ON FIELDS - Sharing Internal Representations in Indexed Family Containers
37