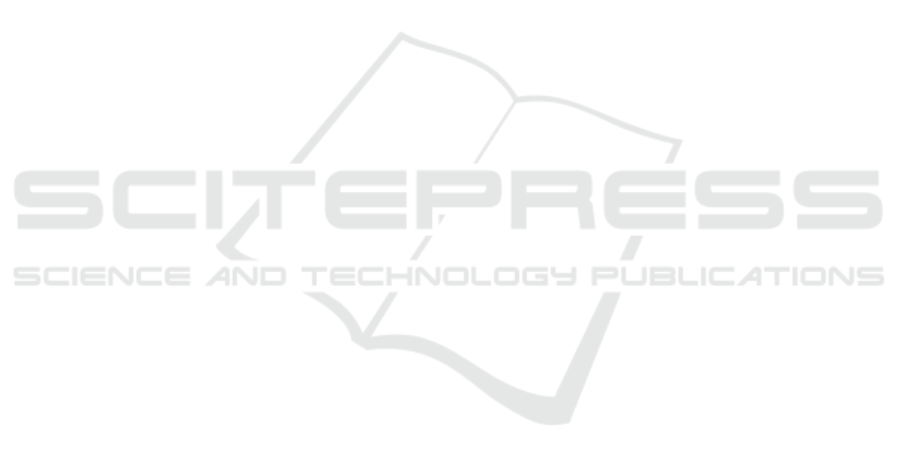
France, August 28-31, 2007, Proceedings, pages 72–
81.
Cole, M. (1991). Algorithmic Skeletons: Structured Man-
agement of Parallel Computation. MIT Press, Cam-
bridge, MA, USA.
Cooley, J. and Tukey, J. (1965). An algorithm for the ma-
chine calculation of complex fourier series. Mathe-
matics of Computation, 19(90):297–301.
Cosmo, R. D., Li, Z., Pelagatti, S., and Weis, P. (2008).
Skeletal Parallel Programming with OcamlP3l 2.0.
18(1):149–164.
Danelutto, M. and Torquati, M. (2015). Structured paral-
lel programming with ”core” fastflow. Central Euro-
pean Functional Programming School. CEFP 2013.
Lecture Notes in Computer Science, 8606:29–75.
Di Cosmo, R. and Danelutto, M. (2012). A “minimal dis-
ruption” skeleton experiment: seamless map & reduce
embedding in OCaml. In International Conference
on Computational Science (ICCS), volume 9, pages
1837–1846. Elsevier.
Falcou, J., S
´
erot, J., Chateau, T., and Laprest
´
e, J.-T. (2006).
Quaff: Efficient C++ Design for Parallel Skeletons.
Parallel Computing, 32:604–615.
Gamma, E., Helm, R., Johnson, R., and Vlissides, J.
(1995). Design Patterns: Elements of Reusable
Object-oriented Software. Addison-Wesley Longman
Publishing Co., Inc., Boston, MA, USA.
Hammond, K. and Portillo,
´
A. J. R. (1999). Haskskel: Al-
gorithmic skeletons in haskell. In IFL, volume 1868
of LNCS, pages 181–198. Springer.
Intel (2019). Intel MPI library developer reference for
Linux OS: Java bindings for MPI-2 routines. Ac-
cessed: 20-November-2019.
Javed, A., Qamar, B., Jameel, M., Shafi, A., and Carpen-
ter, B. (2016). Towards scalable Java HPC with hy-
brid and native communication devices in MPJ Ex-
press. International Journal of Parallel Programming,
44(6):1142–1172.
Kornerup, J. (1997). Data Structures for Parallel Recursion.
Ph.d. dissertation, University of Texas.
L
´
egaux, J., Loulergue, F., and Jubertie, S. (2013). OSL:
an algorithmic skeleton library with exceptions. In
International Conference on Computational Science
(ICCS), pages 260–269, Barcelona, Spain. Elsevier.
Leyton, M. and Piquer, J. M. (2010). Skandium: Multi-
core programming with algorithmic skeletons. In
18th Euromicro Conference on Parallel, Distributed
and Network-based Processing (PDP), pages 289–
296. IEEE Computer Society.
Loulergue, F. and Philippe, J. (2019). Automatic Opti-
mization of Python Skeletal Parallel Programs. In
Algorithms and Architectures for Parallel Processing
(ICA3PP), LNCS, pages 183–197, Melbourne, Aus-
tralia. Springer.
Marlow, S., editor (2010). Haskell 2010 Language Report.
Minsky, Y. (2011). OCaml for the masses. Communications
of the ACM, 54(11):53–58.
Misra, J. (1994). Powerlist: A structure for parallel recur-
sion. ACM Trans. Program. Lang. Syst., 16(6):1737–
1767.
MPI. Mpi: A message-passing interface standard. https://
www.mpi-forum.org/docs/mpi-3.1/mpi31-report.pdf.
Accessed: 20-November-2019.
Niculescu, V., Bufnea, D., and Sterca, A. (2019). MPI scal-
ing up for powerlist based parallel programs. In 27th
Euromicro International Conference on Parallel, Dis-
tributed and Network-Based Processing, PDP 2019,
Pavia, Italy, February 13-15, 2019, pages 199–204.
IEEE.
Niculescu, V. and Loulergue., F. (2018). Transform-
ing powerlist based divide&conquer programs for an
improved execution model. In High Level Paral-
lel Programming and Applications (HLPP), Orleans,
France.
Niculescu, V., Loulergue, F., Bufnea, D., and Sterca, A.
(2017). A Java framework for high level paral-
lel programming using powerlists. In 18th Interna-
tional Conference on Parallel and Distributed Com-
puting, Applications and Technologies, PDCAT 2017,
Taipei, Taiwan, December 18-20, 2017, pages 255–
262. IEEE.
Oracle. The Java tutorials: ForkJoinPool.
https://docs.oracle.com/javase/tutorial/essential/
concurrency/forkjoin.html. Accessed: 20-November-
2019.
Pelagatti, S. (1998). Structured Development of Parallel
Programs. Taylor & Francis.
Philippe, J. and Loulergue, F. (2019). PySke: Algorith-
mic skeletons for Python. In International Confer-
ence on High Performance Computing and Simulation
(HPCS), pages 40–47. IEEE.
Qamar, B., Javed, A., Jameel, M., Shafi, A., and Carpen-
ter, B. (2014). Design and implementation of hybrid
and native communication devices for Java HPC. In
Proceedings of the International Conference on Com-
putational Science, ICCS 2014, Cairns, Queensland,
Australia, 10-12 June, 2014, pages 184–197.
Vega-Gisbert, O., Rom
´
an, J. E., and Squyres, J. M. (2016).
Design and implementation of Java bindings in Open
MPI. Parallel Computing, 59:1–20.
Veldhuizen, T. (2000). Techniques for Scientific C++. Com-
puter science technical report 542, Indiana University.
Pattern-driven Design of a Multiparadigm Parallel Programming Framework
61