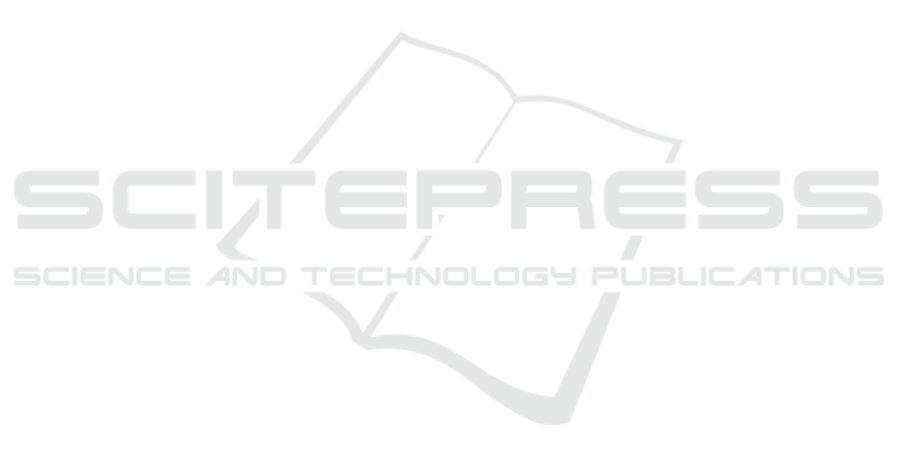
blocks.
In the actor model, if the result of failing any secu-
rity check is to recover to the starting state, there can
be a single supervisor and one actor for each security
check, i.e. n + 1 code blocks. Of course, more elabo-
rate recovery strategies are possible, and will typically
generate more code blocks.
6 SUMMARY AND FUTURE
WORK
Programming languages support multiple reliability
mechanisms, and these have a key role in securing
web applications. This paper seeks to provide in-
formation to guide Scala developers on what mech-
anisms to select. Our results are based on measure-
ments of three instances of a simple secure web app
using the popular Scala/Akka platform, and the key
findings can be summarised as follows.
Performance. (1) All reliability mechanisms fail fast:
unsuccessful requests have low mean latency(1-2ms
in Figure 3) but dramatically reduce throughput: by
more than 100x in Figure 2. (2) For a realistic au-
thentication workloads exceptions have the highest
throughput (187K req/s) and the lowest mean latency
(around 5ms), followed by futures (156K req/s; 6ms)
and actors (108K req/s; 10ms) (Figures 4 and 5).
Programmability. For authentication and authorisa-
tion, actors have the smallest number of code blocks
both for our benchmark (and for a sequence of n secu-
rity checks) namely 3 (n+ 1), and both futures and ex-
ceptions have 4 (2n) code blocks (Figure 6). We con-
clude that Actors minimise programming complexity
and hence attack surface.
Recommendations. Our Scala study reveals an
inverse relationship between performance and pro-
grammability. So for Scala when throughput and la-
tency are not critical, security is paramount, or un-
successful requests are frequent, actors are the best
choice as they minimise complexity and reduce attack
surface. Exceptions provide better performance at the
cost of greater complexity and attack surface. Futures
occupy a middle ground between exceptions and ac-
tors.
Ongoing Work. The current study can be extended in
a number of ways. For example, currently, there are
a fixed number of supervisors in the actor implemen-
tation (10). For workloads with varying failure rates,
we could investigate whether raising or lowering the
number of supervised actors improves throughput and
latency.
Longer term goals are to investigate other applica-
tion domains, and beyond Scala/Akka. For example,
Erlang has far more lightweight actors and may de-
liver very different performance. Likewise, futures
might have better performance in a functional lan-
guage, such as Haskell.
REFERENCES
Bagwell (2010). Scala at LinkedIn — The Scala
Programming Language. https://www.scala-
lang.org/old/node/6436. Accessed: 23-03-2019.
Deepak Vasthimal 2016 (2016). Scalable and Nimble Con-
tinuous Integration for Hadoop Projects. Accessed:
23-03-2019.
Dony, C., Knudsen, J. L., and et al (2006). Advanced Topics
in Exception Handling Techniques. Springer-Verlag,
Berlin, Heidelberg.
Errors are values - The Go Blog (2015). Errors are val-
ues - The Go Blog. https://blog.golang.org/errors-are-
values. Accessed: 05-09-2020.
European Union (2016). Regulation (EU) 2016/679 of the
European Parliament. Official Journal of the Euro-
pean Union, L119:1–88.
Fenton, N. and Bieman, J. (2014). Software metrics: a rig-
orous and practical approach. CRC press.
Hewitt, C., Bishop, P., and Steiger, R. (1973). A univer-
sal modular actor formalism for artificial intelligence.
In Advance Papers of the Conference, volume 3 of IJ-
CAI’73, page 235, Stanford, USA. Stanford Research
Institute.
Kissel, R. (2013). Nist ir 7298 revision 2: Glossary of key
information security terms. National Institute of Stan-
dards and Technology, 7.
Klabnik, S. and Nichols, C. (2019). The Rust Programming
Language (Covers Rust 2018). No Starch Press.
Liskov, B. and Shrira, L. (1988). Promises: Linguistic sup-
port for efficient asynchronous procedure calls in dis-
tributed systems. SIGPLAN Not., 23(7).
Mare, S., Baker, M., and Gummeson, J. (2016). A study of
authentication in daily life. In Twelfth Symposium on
Usable Privacy and Security ({SOUPS} 2016).
Marius Eriksen 2012 (2012). Scala at Twitter. Accessed:
23-03-2019.
McCabe, T. J. (1976). A complexity measure. IEEE Trans.
Softw. Eng., 2(4).
Miller, J. A., Bowman, C., Harish, V. G., and Quinn, S.
(2016). Open Source Big Data Analytics Frame-
works Written in Scala. In 2016 IEEE International
Congress on Big Data (BigData Congress).
Moshtari, S., Sami, A., and Azimi, M. (2013). Using com-
plexity metrics to improve software security. Com-
puter Fraud & Security, 5.
Odersky, M., Spoon, L., and Venners, B. (2008). Program-
ming in Scala. Artima Inc.
Penev, D. (2019a). Comparing reliability mechanisms for
secure web servers. BSc Thesis, Glasgow University.
Comparing Reliability Mechanisms for Secure Web Servers: Comparing Actors, Exceptions and Futures in Scala
57