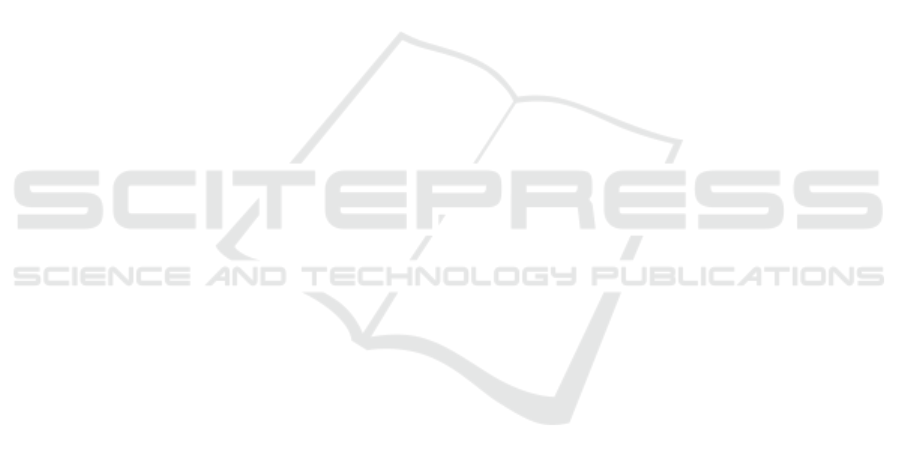
7 CONCLUSIONS
In this paper, we identified a problem that makes it
hard to use unit testing in conjunction with aspect-
oriented code. The problem is that unit tests in AOP
systems must always test the system after the advises
from any modules containing aspects have been ap-
plied. If these aspects are invasive, then the tests are
not testing the unit in isolation and they stop being
unit tests.
The solution we proposed is based on having tests,
that test modules that contain invasive aspects, anno-
tated in such a way that they announce which tests
test the functionality being modified by those aspects.
Having these annotations in place would allow a test-
ing technique based on incremental compilation that
could test units in lower layers of the software, using
their own unit tests, separately from invasive aspects
from higher layers. We argued that this is similar to
what stubs and mocks contributed to object-oriented
unit testing.
We do not argue that the proposed solution is us-
able in every situation, but we have shown that it can
be used in several different scenarios. We envision it
being used in software houses that have a large repos-
itory of modules that can be combined in different
ways in order to compose different software solutions.
Anyone that has tried to create such a system knows
that crosscutting concerns are a big issue.
ACKNOWLEDGEMENTS
We would like to thank FCT for the support provided
through scholarship SFRH/BD/32730/2006.
REFERENCES
Assunção, W. K. G., Colanzi, T. E., Vergilio, S. R., and
Ramirez Pozo, A. T. (2014). Evaluating different
strategies for integration testing of aspect-oriented
programs. Journal of the Brazilian Computer Society,
20(1).
Balzarotti, D. and Monga, M. (2004). Using program
slicing to analyze aspect-oriented composition. In
Proceedings of Foundations of Aspect-Oriented Lan-
guages Workshop at AOSD, pages 25–29.
Baniassad, E. and Clarke, S. (2004). Theme: An approach
for aspect-oriented analysis and design. In Proceed-
ings of the 26th International Conference on Software
Engineering, pages 158–167. IEEE Computer Soci-
ety.
Ceccato, M., Tonella, P., and Ricca, F. (2005). Is AOP
Code Easier to Test than OOP Code? In Work-
shop on Testing Aspect-Oriented Programs, Interna-
tional Conference on Aspect-Oriented Software De-
velopment, Chicago, Illinois.
Fowler, M. (2007). Mocks aren’t stubs. Online article at
martinfowler.com http://bit.ly/18BPLE1.
Greenwood, P., Garcia, A. F., Bartolomei, T., Soares, S.,
Borba, P., and Rashid, A. (2007). On the design of
an end-to-end aosd testbed for software stability. In
ASAT: Proceedings of the 1st International Workshop
on Assessment of Aspect-Oriented Technologies, Van-
couver, Canada. Citeseer.
Gregor Kiczales, John Lamping, Anurag Mendhekar, Chris
Maeda, Cristina Lopes, Jean-Marc Loingtier, and
John Irwin (1997). Aspect-Oriented Programming.
Proceedings of the European Conference on Object-
Oriented Programming, 1241:220–242.
Havinga, W., Nagy, I., Bergmans, L., and Aksit, M. (2007).
A graph-based approach to modeling and detecting
composition conflicts related to introductions. In
AOSD: Proceedings of the 6th International Con-
ference on Aspect-Oriented Software Development,
pages 85–95, New York, NY, USA. ACM Press.
Katz, S. (2004). Diagnosis of harmful aspects using regres-
sion verification. In FOAL: Foundations Of Aspect-
Oriented Languages, pages 1–6.
Kienzle, J., Yu, Y., and Xiong, J. (2003). On composition
and reuse of aspects. In SPLAT: Software Engineering
Properties of Languages for Aspect Technologies.
Lagaisse, B., Joosen, W., and De Win, B. (2004). Manag-
ing semantic interference with aspect integration con-
tracts. In SPLAT: Software Engineering Properties of
Languages for Aspect Technologies.
Marius Marin, L. M. and van Deursen, A. (2007). An inte-
grated crosscutting concern migration strategy and its
application to jhotdraw. Technical report, Delft Uni-
versity of Technology Software Engineering Research
Group.
Peri Tarr, Harold Ossher, William Harrison, and Stanley M.
Sutton Jr. (1999). N degrees of separation: multi-
dimensional separation of concerns. Proceedings of
the International Conference on Software Engineer-
ing, page 107.
Rashid, A., Moreira, A., and Araújo, J. (2003). Modulari-
sation and composition of aspectual requirements. In
Proceedings of the 2nd international conference on
Aspect-oriented software development, pages 11–20.
ACM.
Restivo, A. (2009). DrUID: Unexpected interactions detec-
tion. https://github.com/arestivo/druid.
Restivo, A. (2010). Aida: Automatic interference detection
for aspectj. https://github.com/arestivo/aida.
Restivo, A. (2014). School-aspectj-testbed. https://
github.com/arestivo/School-AspectJ-Testbed.
Restivo, A. and Aguiar, A. (2008). Disciplined composition
of aspects using tests. In LATE: Proceedings of the
2008 AOSD Workshop on Linking Aspect Technology
and Evolution, LATE ’08, pages 8:1–8:5, New York,
NY, USA. ACM.
Restivo, A. and Aguiar, A. (2009). DrUID – unexpected
ICSOFT-PT 2016 - 11th International Conference on Software Paradigm Trends
58