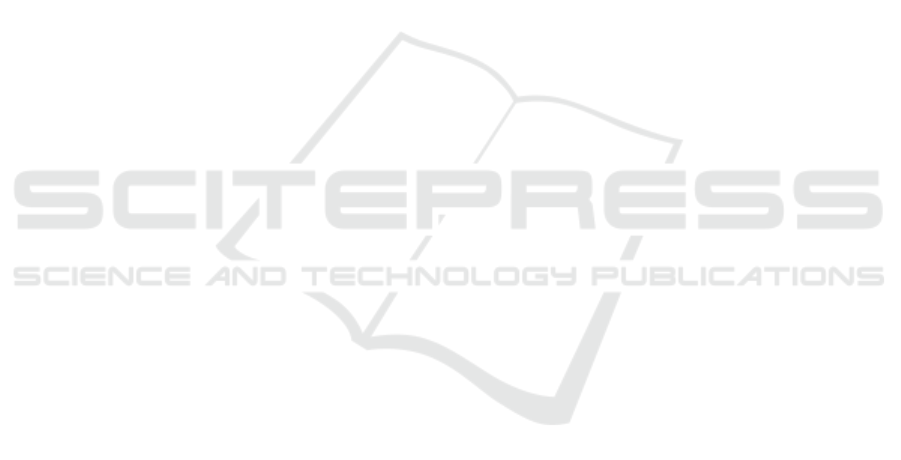
tribution of state. In other words, code does not have
to be propagated through a distributed system along
the same communication paths as data. Consider, for
example, a system where nodes communicate over a
gossip-based protocol. A message might contain se-
rialized data and traverse multiple edges of the gossip
graph before it arrives at a node where the data must
be deserialized. Any intermediate nodes will only be
passing along the serialized data and may never have
a need for the associated code. But the sender does
not know if the target node has the requisite code in-
stalled. So to be safe, the sender will have to include
the possibly redundant code as part of its outgoing
message, or the design must be complicated in some
other way, for example by adding additional rounds
of gossip to retrieve the code.
With the separation of concerns that LADY of-
fers, the design of such gossip-based systems could be
simplified, since code would be retrieved on demand
via an entirely independent mechanism whenever data
was deserialized. Our satellite execution refactoring
in section 4 also helps to illustrate how LADY can
simplify the design of other distributed systems, to
improve extensibility and serve as a convenient foun-
dation for mobile code.
REFERENCES
Semantic Versioning. http://semver.org/.
Cook, R. P. and Lee, I. (1983). DYMOS: A dynamic modi-
fication system. volume 8, pages 201–202, New York,
NY, USA. ACM.
Daubert, E., Fouquet, F., Barais, O., Nain, G., Sunye, G.,
Jezequel, J.-M., Pazat, J.-L., and Morin, B. (2012). A
models@runtime framework for designing and man-
aging service-based applications. In Software Services
and Systems Research - Results and Challenges (S-
Cube), 2012 Workshop on European, pages 10–11.
Dean, J. and Ghemawat, S. (2004). MapReduce: Simplified
data processing on large clusters. In Proceedings of
the 6th symposium on Operating Systems Design and
Implementation, OSDI ’04, pages 137–150. USENIX
Association.
DeCandia, G., Hastorun, D., Jampani, M., Kakulapati,
G., Lakshman, A., Pilchin, A., Sivasubramanian, S.,
Vosshall, P., and Vogels, W. (2007). Dynamo: Ama-
zon’s highly available key-value store. In Proceedings
of the 21st ACM SIGOPS Symposium on Operating
Systems Principles, SOSP ’07, pages 205–220. ACM.
Gilmore, S., Kirli, D., and Walton, C. (1997). Dynamic ML
without dynamic types. Technical report, University
of Edinburgh.
Gkantsidis, C., Karagiannis, T., Rodriguez, P., and Vo-
jnovi
´
c, M. (2006). Planet scale software updates.
ACM SIGCOMM Computer Communication Review,
36(4):423–434.
Hertzog, R. and Mas, R. (2006). The Debian Adminis-
trator’s Handbook. Freexian SARL, https://debian-
handbook.info/, first edition.
Hicks, M., Moore, J. T., and Nettles, S. (2001). Dynamic
software updating. In Proceedings of the ACM SIG-
PLAN 2001 Conference on Programming Language
Design and Implementation, PLDI ’01, pages 13–23,
New York, NY, USA. ACM.
Johansen, D., Lauvset, K. J., van Renesse, R., Schneider,
F. B., Sudmann, N. P., and Jacobsen, K. (2001). A
TACOMA retrospective. Software - Practice and Ex-
perience, 32:605–619.
Johansen, H. and Johansen, D. (2008). Resilient software
mirroring with untrusted third parties. In Proceedings
of the 1st ACM workshop on hot topics in software
upgrades (HotSWUp).
Johansen, H., Johansen, D., and van Renesse, R. (2007).
Firepatch: secure and time-critical dissemination of
software patches. In Proceedings of the 22nd IFIP
International Information Security Conference, pages
373–384. IFIP.
Microsoft (2015). Application Do-
mains. http://msdn.microsoft.com/en-
us/library/cxk374d9%28v=vs.90%29.aspx.
Microsoft Developer Network (2016). Best Practices for
Assembly Loading. Microsoft, .NET Framework
4.6 and 4.5 edition. https://msdn.microsoft.com/en-
us/library/dd153782(v=vs.110).aspx.
Olston, C., Reed, B., Srivastava, U., Kumar, R., and
Tomkins, A. (2008). Pig latin: a not-so-foreign lan-
guage for data processing. In Proceedings of the 2008
ACM SIGMOD international conference on Manage-
ment of data, SIGMOD ’08, pages 1099–1110. ACM.
Pettersen, R., Valvåg, S. V., Kvalnes, A., and Johansen,
D. (2014). Jovaku: Globally distributed caching for
cloud database services using DNS. In IEEE Interna-
tional Conference on Mobile Cloud Computing, Ser-
vices, and Engineering, pages 127–135.
Pettersen, R., Valvåg, S. V., Kvalnes, A., and Johansen, D.
(2015). Cloud-side execution of database queries for
mobile applications. In CLOSER 2015 : Proceedings
of the 5th International Conference on Cloud Comput-
ing and Services Science, pages 586–594.
Raemaekers, S., van Deursen, A., and Visser, J. (2014). Se-
mantic versioning versus breaking changes: A study
of the maven repository. In Source Code Analysis and
Manipulation (SCAM), 2014 IEEE 14th International
Working Conference on, pages 215–224.
Segal, M. and Frieder, O. (1993). On-the-fly program mod-
ification: systems for dynamic updating. Software,
IEEE, 10(2):53–65.
Valvåg, S. V., Johansen, D., and Kvalnes, A. (2013).
Cogset: A high performance MapReduce engine.
Concurrency and Computation: Practice and Expe-
rience, 25(1):2–23.
Yu, Y., Isard, M., Fetterly, D., Budiu, M., Erlingsson, Ú.,
Gunda, P. K., and Currey, J. (2008). DryadLINQ:
A system for general-purpose distributed data-parallel
computing using a high-level language. In Proceed-
ings of the 8th USENIX conference on Operating Sys-
LADY: Dynamic Resolution of Assemblies for Extensible and Distributed .NET Applications
127