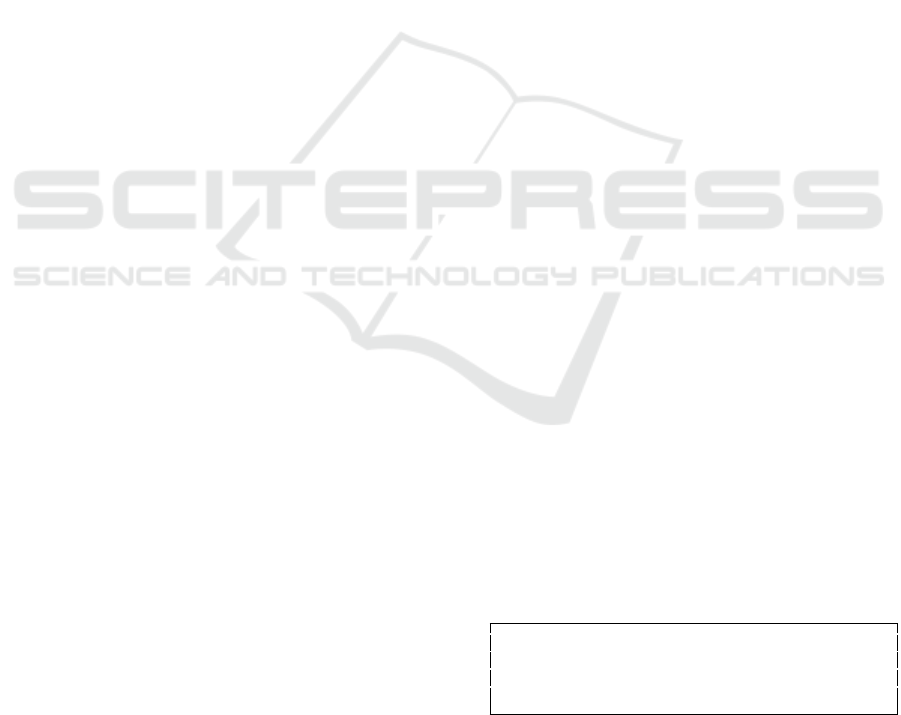
2015), which is a set of 90 small programs in C lan-
guage that novice programmers often mistake.
The result is shown in Table. 2; out of 90, there
are 39 false-positives and 6 true-positives. This result
shows that as we expected, our heuristic approach in-
creases false-positives. The main reasons are because
the current C-Helper supports only 15 errors (see Ap-
pendix), and also because the benchmark includes
many complex expressions that the current C-Helper
cannot handle like if((p=malloc(512))!=NULL)·· ·.
We have a plan to extend and refine our heuristic rules
to decrease false-positives while preserving the merits
of C-Helper.
5 CONCLUSIONS
In this paper, we proposed a novel C static checker
called C-Helper, that aims to emit more direct error
messages understandable for novices to correct wrong
programs, and also aims to handle latent errors. Our
preliminary evaluation shows that C-Helper was pos-
itively evaluated, although our heuristic approach in-
creased false-positives.
As future work, we have a plan to extend and
refine our heuristic rules to decrease false-positives
while preserving the merits of C-Helper.
REFERENCES
Flowers, T., Carver, C., and Jackson, J. (2004). Empow-
ering students and building confidence in novice pro-
grammers through gauntlet. In Frontiers in Education,
2004. FIE 2004. 34th Annual, pages T3H/10–T3H/13
Vol. 1.
Freund, S. N. and Roberts, E. S. (1996). Thetis: An ansi c
programming environment designed for introductory
use. In Proceedings of the Twenty-seventh SIGCSE
Technical Symposium on Computer Science Educa-
tion, SIGCSE ’96, pages 300–304, New York, NY,
USA. ACM.
Hristova, M., Misra, A., Rutter, M., and Mercuri, R. (2003).
Identifying and correcting java programming errors
for introductory computer science students. SIGCSE
Bull., 35(1):153–156.
Inexpensive Program Analysis Group, University of Vir-
ginia, D. o. C. S. (2015). Splint annotation-assisted
lightweight static checking. http://www.splint.org/,
[Online; accessed 14-Oct-2015].
K
¨
olling, M., Quig, B., Patterson, A., and Rosenberg, J.
(2003). The bluej system and its pedagogy. Computer
Science Education, 13(4):249–268.
Kummerfeld, S. K. and Kay, J. (2003). The neglected battle
fields of syntax errors. In Proceedings of the Fifth Aus-
tralasian Conference on Computing Education - Vol-
ume 20, ACE ’03, pages 105–111, Darlinghurst, Aus-
tralia, Australia. Australian Computer Society, Inc.
Marceau, G., Fisler, K., and Krishnamurthi, S. (2011a).
Measuring the effectiveness of error messages de-
signed for novice programmers. In Proceedings of the
42Nd ACM Technical Symposium on Computer Sci-
ence Education, SIGCSE ’11, pages 499–504, New
York, NY, USA. ACM.
Marceau, G., Fisler, K., and Krishnamurthi, S. (2011b).
Mind your language: On novices’ interactions with
error messages. In Proceedings of the 10th SIG-
PLAN Symposium on New Ideas, New Paradigms, and
Reflections on Programming and Software, Onward!
2011, pages 3–18, New York, NY, USA. ACM.
Nielson, F., Nielson, H. R., and Hankin, C. (2004). Princi-
ples of Program Analysis. Springer; Corrected edition.
Osuka, T., Kobayashi, T., Atsumi, N., Mase, J., Ya-
mamoto, S., Suzumura, N., and Agusa, K. (2012).
CX-checker: A flexibly customizable coding checker
for C. Journal of Information Processing Society of
Japan, 53(2):590–600.
RemicalSoft (2015). Forum of any ques-
tions in the programming language C.
http://dixq.net/forum/viewforum.php?f=3, [Online;
accessed 14-Oct-2015].
Song, J. S., Hahn, S. H., Tak, K. Y., and Kim, J. H. (1997).
An intelligent tutoring system for introductory c lan-
guage course. Comput. Educ., 28(2):93–102.
uchan-nos (2015). C-helper: A programming environ-
ment for beginners of C programming language.
https://github.com/uchan-nos/c-helper, [Online; ac-
cessed 14-Oct-2015].
Yoshitaka Kojima, Yoshitaka Arahori, K. G. (2015). Inves-
tigating the difficulty of commercial-level compiler
warning messages for novice programmers. In Pro-
ceedings of the 8th International Conference on Com-
puter Supported Education, CSEDU! 2015, pages
483–490.
APPENDIX
The following is the list of 15 errors checked by the
current C-Helper, categorized into three groups: (1) 2
syntax errors, (2) 6 semantic errors, (3) 7 latent errors.
Listing 5: Example of extra semicolon in function defini-
tion.
1 #include <stdi o .h>
2 int main (void ) ;
3 {
4 }
Syntax Errors
E1: Extra semicolon in function definition
CSEDU 2016 - 8th International Conference on Computer Supported Education
326