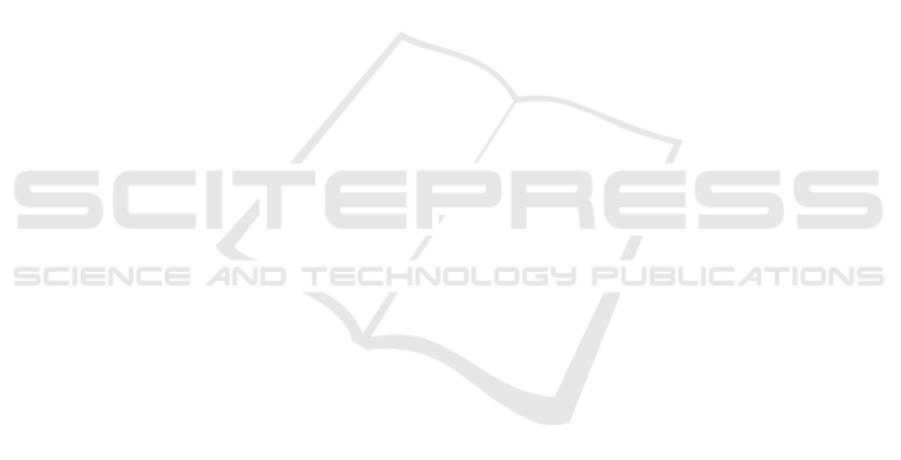
An EMF-like UML Generator for C++
Sven J
¨
ager, Ralph Maschotta, Tino Jungebloud, Alexander Wichmann and Armin Zimmermann
Systems and Software Engineering Group, Computer Science and Automation Department,
Technische Universit
¨
at Ilmenau, Ilmenau, Germany
Keywords:
Model based Software Development, Code Generation, Meta Modeling, C++, UML, MOF, Ecore.
Abstract:
Model-driven architecture is a well-known approach for the development of complex software systems. The
most famous tool chain is provided by Eclipse with the tools of the Eclipse modeling project. Like Eclipse
itself, these tools are based on Java. However, there are numerous legacy software packages written in C++,
which often use only an implicit meta-model. A real C++ implementation of this meta-model would be
necessary instead to be used at run time. This paper presents a generator for C++ to create the classes,
meta-model packages, and factories to realize modeling, transformation, validation, and comparison of UML
models. It gives an overview of its workflow and major challenges. Moreover, a comparison between Java and
C++ implementations is given, considering different benchmarks.
1 INTRODUCTION
The Model Driven Architecture (MDA) approach as
defined by the Object Management Group (OMG)
is a well-known family of standards which unifies
every step of the development of an application
from Platform-Independent Model (PIM) through
Platform-Specific Models (PSM) to generated code
and a deployable application (OMG, 2014). The
common Eclipse Modeling Project (EMP) supports
this approach by providing a unified set of model-
ing frameworks, tool support, and standard imple-
mentations based on the Eclipse Modeling Frame-
work (EMF) (Steinberg et al., 2009; Gronback, 2009).
It uses Ecore, a meta meta-model which is similar
to the essential meta object facility (EMOF) for de-
scribing a meta-model. The EMF framework with its
code generation facility can be used to build tools and
other applications based on a structured data model,
like complete editors for Ecore-based domain specific
meta-models. It produces Java classes for the model
and other necessary utilities to create and edit such
a model. Therefore, the MDA approach is well inte-
grated with the Java programming language, but it is
weakly supported for other programming languages
such as C++.
C++ is one of the most commonly used program-
ming languages (TIOBE Software BV, 2015). Nu-
merous legacy software packages are written in C++
and use often only an implicit meta-model. To use a
real meta-model at runtime, an implementation of the
meta-model in C++ would be necessary (Jungebloud
et al., 2013). Such a C++ meta-model could be
used, for instance, to configure dialog properties at
runtime, to realize a runtime Object Constraint Lan-
guage (OCL) (OMG, 2012) for the checking of model
elements, or to execute a behavior which is described
by activity diagrams.
There are mainly two possibilities to create such
meta-model representations. The obvious one is
to write code and implement every class manually.
When the number of elements in a model increases,
however, the best way to overcome the implementa-
tion is to use a generator. A big advantage of a gen-
erator (besides saving implementation time) is the de-
terministic result of the transformation. Each model
element is transformed in the same way and produces
similar code blocks. By using guidelines like the
MISRA C++ (MISRA, 2008) for the generator, the
resulting code can be used in safety-critical software.
EMF4CPP (Gonz
´
alez et al., 2010) is an avail-
able implementation of a C++ Ecore meta-model and
comes with a generator for Ecore to C++ transfor-
mation. Preliminary results show that “. . . memory
consumption and efficiency is usually better in
EMF4CPP than in Java. . . ” (EMF4Cpp, 2015). Un-
fortunately, the last visible development activities in
this project date back to the year 2011.
Moreover, this generator only provides the possi-
bility to transform Ecore models to C++ code. The
transformation of the more expressive UML (unified
modeling language) (OMG, 2015b) is not considered.
A native generator for C++ is necessary to use all
capabilities of UML in describing the structure and
Jäger, S., Maschotta, R., Jungebloud, T., Wichmann, A. and Zimmermann, A.
An EMF-like UML Generator for C++.
DOI: 10.5220/0005744803090316
In Proceedings of the 4th International Conference on Model-Driven Engineering and Software Development (MODELSWARD 2016), pages 309-316
ISBN: 978-989-758-168-7
Copyright
c
2016 by SCITEPRESS – Science and Technology Publications, Lda. All rights reserved
309