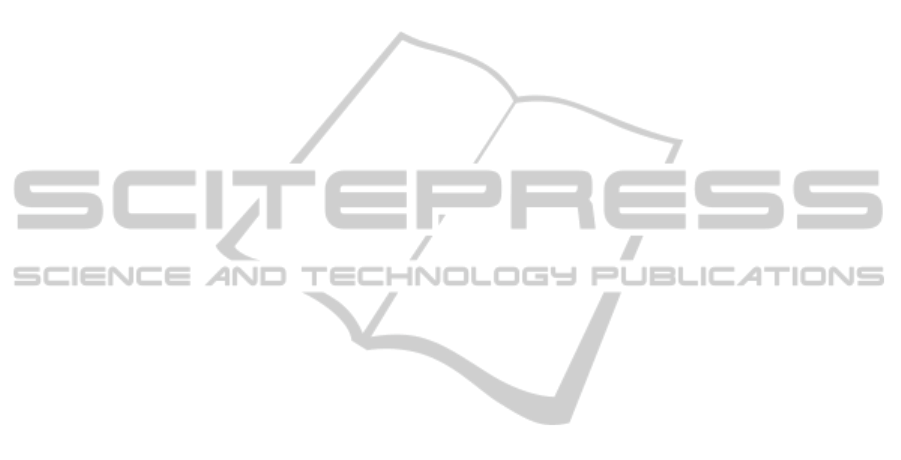
from the root of the tree to the result vertices of an
XPath query can be improved. Therefore two algo-
rithms were proposed. The algorithms were designed
for a relational column-oriented in-memory database
and rely on pre-post-order numbering. The sort-tilt-
scan improves the na¨ıve approach by using two sorted
tables and minimizing the number of rows that have
to be scanned for a vertex to find its ancestors. The
single-pass-scan exploits the fact that the result is a
set and does not need the information how often a
certain vertex was found as an ancestor. In this way
it is possible to solve the problem with a single ta-
ble scan. In the evaluation part it was found that the
single-pass-scan greatly improves the performance of
the task, especially for queries with a high selectivity.
It was significantly faster than other algorithms, re-
gardless of the database size, the queries selectivity
or the distribution of the results.
Since the single-pass-scan is based on a table
scan, it provides possibilities for parallelization. Fur-
ther tests should show how the algorithms behave in
a multithreaded environment. Another approach for
fetching the paths to the result vertices would be us-
ing a streaming-based XPath engine as XSQ (Peng
and Chawathe, 2005). It could be modified to keep
a stack of ancestors and write them into the result
once the query produced a hit. However, this might
slow down the actual execution of the query, as the
advantage of targeted, index-based access to the data
could not be used. Finally, it should be mentioned
that with a slight modification the algorithms could
be used to fetch the descendants of a set of vertices
as well. This way, a new potential for optimizing the
implementation of the XPath axes arises. Thus, the al-
gorithms proposed in this paper can not only improve
the post-processing of the result, but the evaluation of
the query as well.
REFERENCES
Chen, Y., Davidson, S. B., and Zheng, Y. (2004). BLAS:
an efficient XPath processing system. In Proceedings
of the SIGMOD international conference on Manage-
ment of data, pages 47–58, New York, NY, USA.
ACM.
Cockshott, W. P., McGregor, D., Kotsis, N., and Wil-
son, J. (1998). Data Compression in Database Sys-
tems. In Proceedings of the International Workshop
on Database and Expert Systems Applications, page
981. IEEE Computer Society.
Gou, G. and Chirkova, R. (2007). Efficient Algorithms for
Evaluating XPath over Streams. In Proceedings of the
SIGMOD international conference on Management of
data, pages 269–280, New York, NY, USA. ACM.
Grust, T., Keulen, M., and Teubner, J. (2004). Accelerating
XPath evaluation in any RDBMS. ACM Transactions
on Database Systems, 1.
Li, F., Agrawal, P., Eberhardt, G., Manavoglu, E., Ugurel,
S., and Kandemir, M. (2004). Improving Memory
Performance of Embedded Java Applications by Dy-
namic Layout Modifications. In Proceedings of the In-
ternational Parallel and Distributed Processing Sym-
posium, page 159. IEEE Computer Society.
Li, X. and Agrawal, G. (2005). Efficient Evaluation of
XQuery over Streaming Data. In Proceedings of
the 31st international conference on Very large data
bases, pages 265–276. VLDB Endowment.
Panchenko, O., Treffer, A., and Zeier, A. (2010). To-
wards Query Formulation and Visualization of Struc-
tural Search Results. In Proceedings of the ICSE
Workshop on Search-driven Development: Users, In-
frastructure, Tools and Evaluation, pages 33–36, New
York, NY, USA. ACM.
Peng, F. and Chawathe, S. S. (2005). XSQ: A Streaming
XPath Engine. ACM Transactions on Database Sys-
tems, 30(2):577–623.
TWO ALGORITHMS FOR LOCATING ANCESTORS OF A LARGE SET OF VERTICES IN A TREE
285