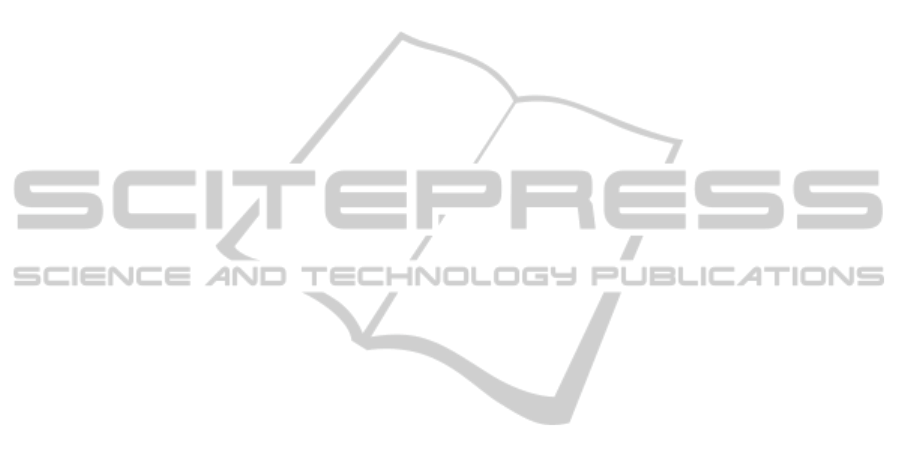
break point. By answering questions about the sound-
ness of some method calls the user can concentrate
on the semantics. A major drawback of declarative
debugging is the fact, that we can only determine a
buggy method call but not the buggy statements in-
side a method. This downside is cured by the fact
that JHyde does also support omniscient debugging.
With omniscient debugging the user can track the ex-
ecution of a debuggee program statement-wise back
and forth in time. The combination of both debug-
ging techniques JHyde can profit from the advantages
both methods, the abstraction of the declarative de-
bugging method and the precision of the omniscient
debugging method.
The JHyde user interface consists of four views
which allow the user to inspect and navigate the exe-
cution history of a debuggee program effectively. At
any point in the debugging process the user is free
to choose the most suitable views and/or debugging
method.
A particular novelty of our approach is the us-
age of code-coverage criteria such as def-use chain
coverage and coverage of the edges of the control-
flow graph to calculate the error probability of method
calls. The error probability increases with the num-
ber of covered entities, i.e. def-use chains or edges
of the CFG. Furthermore, our strategy takes previous
user answers into account. The more often an entity
is classified as valid the lesser its contribution to the
error probability of a method call.
We have conducted a number of tests, using dif-
ferent declarative debugging strategies to debug a set
of buggy Java programs. The results show that up
to 40% of the questions asked can be saved with our
coverage-based D&Q strategy w.r.t. the ordinary top-
down strategy. Furthermore, our strategy is an effi-
cient improvement to the ordinary D&Q strategy. In
our test scenario the number of questions saved could
be nearly doubled if D&Q is enhanced by coverage-
based error probability. The improved reduction of
the debugging effort makes our debugger more suit-
able for real-world applications where the debugging
effort has great influences on the costs of the software
development process.
In the future we plan to investigate how our hybrid
debugging method can be extended to multi-threaded
program executions. Although, our debugger is al-
ready capable of recording multi-threaded programs,
there are still some problems to be tackled regarding a
multi-threaded hybrid debugging method. For exam-
ple, it is much harder to check the validity of method
calls if their execution is interleaved.
REFERENCES
Caballero, R., Hermanns, C., and Kuchen, H. (2007). Al-
gorithmic Debugging of Java Programs. Electronic
Notes in Theoretical Computer Science, 177:75–89.
Caballero, R. and Rodr´ıguez-Artalejo, M. (2002). A Declar-
ative Debugging System for Lazy Functional Logic
Programs. Electronic Notes in Theoretical Computer
Science, 64.
Cormen, T. H., Leiserson, C. E., Rivest, R. L., and Stein, C.
(2001). Introduction to Algorithms. The MIT Press,
2nd edition.
Eclipse Foundation (2010). Eclipse IDE. http://
www.eclipse.org/.
Fritzson, P., Shahmehri, N., Kamkar, M., and Gyimothy, T.
(1992). Generalized algorithmic debugging and test-
ing. ACM Letters on Programming Languages and
Systems, 1(4):303–322.
Gamma, E., Helm, R., Johnson, R., and Vlissides, J. (1995).
Design Patterns. Addison-Wesley, Boston, MA.
Girgis, H. Z. and Jayaraman, B. (2006). JavaDD: a Declara-
tive Debugger for Java. Technical report, Department
of Computer Science and Engineering, University at
Buffalo.
Hailpern, B. and Santhanam, P. (2002). Software debug-
ging, testing, and verification. IBM Systems Journal,
41(1):4–12.
Hermanns, C. (2010). JHyde - Eclipse plugin. http://
www.wi.uni-muenster.de/pi/personal/hermanns.php.
Kouh, H.-J., Kim, K.-T., Jo, S.-M., and Yoo, W.-H. (2004).
Computational Science and Its Applications - ICCSA
2004, volume 3046 of Lecture Notes in Computer
Science, chapter Debugging of Java Programs Using
HDT with Program Slicing, pages 524–533. Springer.
Lewis, B. (2003). Debugging Backwards in Time. CoRR,
cs.SE/0310016.
Nilsson, H. (2001). How to look busy while being as lazy as
ever: the Implementation of a lazy functional debug-
ger. Journal of Functional Programming, 11(6):629–
671.
Object Web (2009). Asm. http://asm.ow2.org/.
Pressman, R. S. (2001). Software Engineering: A Practi-
tioner’s Approach. McGrap-Hill, fifth edition.
Shahmehri, N. and Fritzson, P. (1991). Compiler Compil-
ers, volume 477 of Lecture Notes in Computer Sci-
ence, chapter Algorithmic debugging for imperative
languages with side-effects, pages 226–227. Springer.
Shapiro, E. Y. (1983). Algorithmic Program DeBugging.
MIT Press.
Silva, J. (2007). Logic-Based Program Synthesis and Trans-
formation, volume 4407 of Lecture Notes in Computer
Science, chapter A Comparative Study of Algorithmic
Debugging Strategies, pages 143–159. Springer.
Zeller, A. (2005). Why Programs Fail: A Guide to System-
atic Debugging, chapter How Failures Come to Be,
pages 1–26. Morgan Kaufmann.
JHYDE - THE JAVA HYBRID DEBUGGER
35