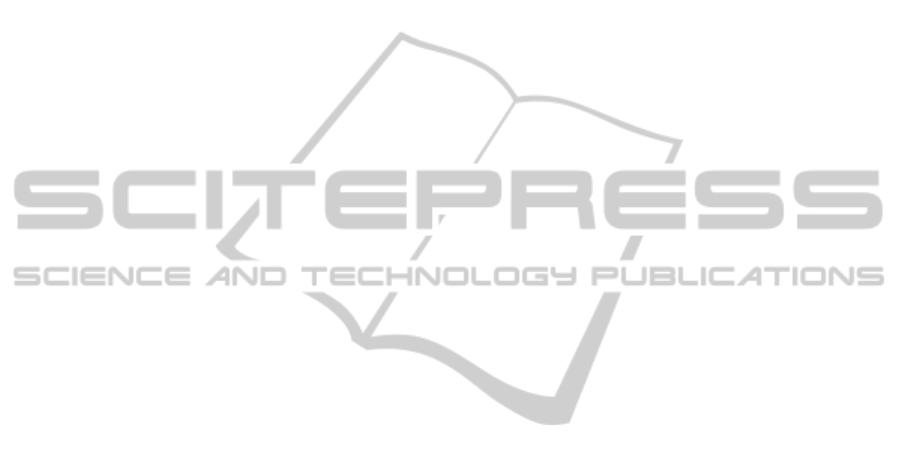
inside existing implementations to enable more effec-
tive verification, but this belongs to our future work.
7 CONCLUSIONS
We present a high-level specification language, iFSM,
to effectively unify the behavior design of object-
oriented classes with detailed implementation strate-
gies. We have automatically generated efficient
C++/Java code from iFSM specifications and have
automatically verified their consistency via model
checking techniques.
REFERENCES
Bagge, O. S., Kalleberg, K. T., Haveraaen, M., and Visser,
E. (2003). Design of the CodeBoost transformation
system for domain-specific optimisation of C++ pro-
grams. In Binkley, D. and Tonella, P., editors, Third
International Workshop on Source Code Analysis and
Manipulation (SCAM 2003), pages 65–75, Amster-
dam, The Netherlands. IEEE Computer Society Press.
Balasubramanian, K., Krishna, A. S., Turkay, E., Balasub-
ramanian, J., Parsons, J., Gokhale, A., and Schmidt,
D. C. (2005). Applying model-driven development to
distributed real-time and embedded avionics systems.
International Journal of Embedded Systems. Special
issue on Design and Verification of Real-time Embed-
ded Software.
Baxter, I., Pidgeon, P., and Mehlich, M. (2004). Dms: Pro-
gram transformations for practical scalable software
evolution. In Proceedings of the International Con-
ference on Software Engineering. IEEE Press.
Beyer, D., Chlipala, A. J., and Majumdar, R. (2004). Gen-
erating tests from counterexamples. In Proceedings of
the 26th International Conference on Software Engi-
neering (ICSE), pages 326–335.
Bravenboer, M., Kalleberg, K. T., Vermaas, R., and Visser,
E. (2008). Stratego/XT 0.17. A language and toolset
for program transformation. Science of Computer
Programming.
Chaki, S., Clarke, E., and Groce, A. (2004). Modular veri-
fication of software components in c. Transactions of
Software Engineering, 1(8).
Cimatti, A. and et. al. (2002). NuSMV Version 2: An Open-
Source Tool for Symbolic Model Checking. In CAV,
volume 2404 of LNCS.
Clarke, E. M., Grumberg, O., and Peled, D. A. (1999).
Model Checking. MIT Press.
Das, M. and et. al. (2002). Esp: path-sensitive program
verification in polynomial time. In PLDI ’02, pages
57–68.
Erwig, M. and Ren, D. (2002). A rule-based language
for programming software updates. SIGPLAN Not.,
37(12):88–97.
Futamura, Y., Konishi, Z., and Gl¨uck, R. (2002). Ws-
dfu: program transformation system based on general-
ized partial computation. The essence of computation:
complexity, analysis, transformation, pages 358–378.
Goguen, J. A. and Burstall, R. M. (1992). Institutions:
abstract model theory for specification and program-
ming. J. ACM, 39(1):95–146.
Gray, J., Bapty, T., and Neema, S. (2001). Handling cross-
cutting constraints in domain-specific modeling. In
Communications of the ACM, pages 87–93.
Harel, D. (1987). Statecharts: A visual formalism for com-
plex systems. Science of Comp. Prog., 8(3).
Harel, D. and Naamad, A. (1996). The statemate seman-
tics of statecharts. ACM Trans. Softw. Eng. Methodol.,
5(4):293–333.
Huang, S. S., Zook, D., and Smaragdakis, Y. (2005). Stat-
ically safe program generation with safegen. In Gen-
erative Programming and Component Engineering.
Kawaguchi, M., Rondon, P., and Jhala, R. (2009). Type-
based data structure verification. In PLDI ’09, pages
304–315.
Kleppe, A., Warmer, J., and Bast, W. (2003). MDA Ex-
plained: The Model Driven Architecture Practice and
Promise. Addison Wesley.
Knapp, A. and Merz., S. (2002). Model checking and code
generation for uml state machines and collaborations.
In Proc. 5th Wsh. Tools for System Design and Verifi-
cation, pages 59–64.
Kogekar, A., Kaul, D., Gokhale, A., Vandal, P., Prapha-
montripong, U., Gokhale, S., Zhang, J., Lin, Y., and
Gray, J. (2006). Model-driven generative techniques
for scalable performability analysis of distributed sys-
tems. In In Proceedings of the NSF NGS Workshop,
International Conference on Parallel and Distributed
Processing Symposium (IPDPS). IEEE.
Levine, J. R., Mason, T., and Brown, D. (1992). Lex & Yacc.
O’Reilly & Associates.
Necula, G. C. (1997). Proof-carrying code. In POPL’97,
pages 106–119.
Niu, J., Atlee, J. M., and Day, N. A. (2003). Template
semantics for model-based notations. IEEE Transac-
tions on Software Engineering, 29(10):866–882.
Owre, S., Rushby, J., and Shankar, N. (1992). PVS: A pro-
totype verification system. In CADE.
Poizat, P., Choppy, C., and Royer, J.-C. (1999). From in-
formal requirements to coop: A concurrent automata
approach. In Proceedings of the Wold Congress on
Formal Methods in the Development of Computing
Systems-Volume II, pages 939–962.
Prout, A., Atlee, J. M., Day, N. A., and Shaker, P. (2008).
Semantically configurable code generation. In MoD-
ELS, pages 705–720.
Wasowski, A. (2003). On efficient program synthesis from
statecharts. In LCTES ’03: Proceedings of the 2003
ACM SIGPLAN conference on Language, compiler,
and tool for embedded systems, pages 163–170, New
York, NY, USA. ACM.
Whalen, M. W. (2000). High-integrity code generation for
state-based formalisms. In ICSE ’00: Proceedings of
the 22nd international conference on Software engi-
neering, pages 725–727, New York, NY, USA. ACM.
Yi, Q., Seymour, K., You, H., Vuduc, R., and Quinlan, D.
(2007). POET: Parameterized optimizations for em-
pirical tuning. In Workshop on Performance Optimiza-
tion for High-Level Languages and Libraries.
ICSOFT 2011 - 6th International Conference on Software and Data Technologies
24