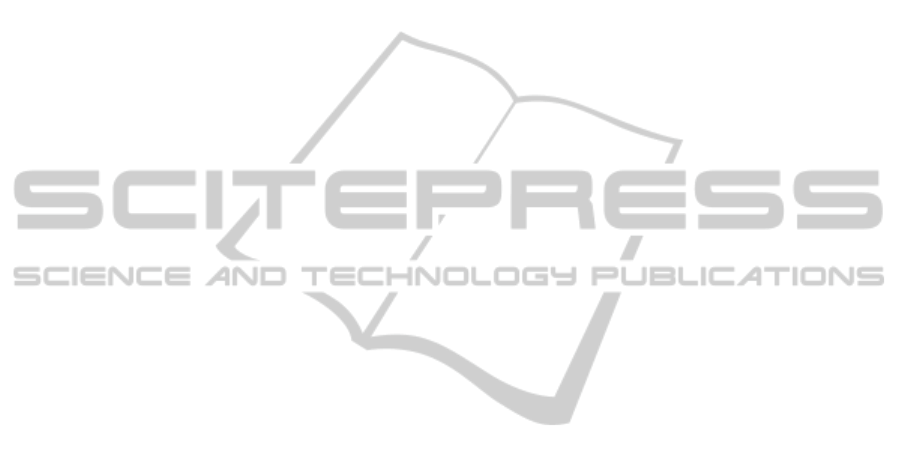
5 CONCLUSIONS
The association of two (or more) parallel hierarchies
to solve a specific problem using delegation is a
common design scenario. The problem is that the
association in the root classes in the hierarchy pro-
vides too general interfaces useless for the classes
below in the hierarchy. The Parallel Hierarchies
design pattern described in this paper provides a
type safe solution that could be used whenever the
programming language provides either F-bound
polymorphism or (unbounded) parametric polymor-
phism such as Java, C# or C++. The design pattern
has been described using the classical sections of
structure, collaborations, consequences, Implemen-
tation, applicability and sample code (Gamma et al.,
1994).
We have successfully used the Parallel Hierar-
chies design pattern in the C# implementation of the
StaDyn programming language (Ortin et al., 2010;
Ortin and Garcia, 2011), to compile the StaDyn
high-level programming language to MSIL for the
CLR, ЯRotor (Redondo, 2008; Ortin et al., 2009)
and the DLR (Hugunin, 2007) platforms, as well as
produce high-level C# 4.0 source code.
ACKNOWLEDGEMENTS
This work has been funded by the Department of
Science and Technology (Spain) under the National
Program for Research, Development and Innovation:
Project TIN2008-00276, entitled Improving Per-
formance and Robustness of Dynamic Languages to
develop Efficient, Scalable and Reliable Software.
REFERENCES
Appel A.W., 2002. Modern Compiler Implementation in
Java, 2nd Edition, Cambridge University Press.
Canning P., Cook W., Hill W., Walter O., and Mitchell J.
C., 1989. F-bounded polymorphism for object-
oriented programming, in Proceedings of the fourth
International Conference on Functional Programming
Languages and Computer Architecture, London, Unit-
ed Kingdom, pp. 273-280.
ECMA 335, 2006. European Computer Manufacturers
Association (ECMA), Common Language Infrastruc-
ture (CLI), Partition IV: CIL Instruction Set, 4th Edi-
tion.
Ernst E., July 2003. Higher-Order Hierarchies. European
Conference on Object-Oriented Programming
(ECOOP), pp. 303-329, Darmstadt, Germany.
Fraser C. W., and Hanson D.R., 1995. A Retargetable C
Compiler: Design and Implemen-tation, Addison-
Wesley Professional.
Fowler M., Beck K., Brant J., Opdyke W., and Roberts D.,
1999. Refactoring: Improving the Design of Existing
Code, Addison-Wesley Professional.
Gamma E., Helm R., Johnson R., and Vlissides J., 1994.
Design Patterns: Elements of Reusable Object-
Oriented Software, Addison Wesley.
Hugunin J., 2007. Bringing dynamic languages to .NET
with the DLR, in Proceedings of the Symposium on
Dynamic Languages, Montreal, Quebec, Canada, pp.
101-101.
JSR 294 Sun Microsystems, 2007. JSR 294: Improved
Modularity Support in the Java Programming Lan-
guage, http://jcp.org/en/jsr/detail?id=294
Lindholm T., and Yellin F., 1999. Java Virtual Machine
Specification, 2nd Edition, Prentice Hall.
Liskov B., 1987. Data Abstraction and Hierarchy, in
Conference on Object Oriented Programming Systems
Languages and Applications (OOPSLA), Orlando,
Florida, United States, pp. 17-34.
Meyer J., Jasmin Instructions, 1996.
http://jasmin.sourceforge.net/instructions.html
Nielsen E.T., Larsen K.A., Markert S., Kjaer K.E., May 31
2005. The Expression Problem in Scala. Technical
Report, Aarhus University.
Odersky M., and Wadler P., 1997. Pizza into Java: Trans-
lating theory into practice, in Proceedings of the 24th
ACM Symposium on Principles of Programming Lan-
guages (POPL), Paris, France, pp. 146-159.
Ortin F., and Garcıa M., 2011. Union and intersection
types to support both dynamic and statict yping. In-
formation Processing Letters, 111(6):278–286.
Ortin F., Redondo J.M., and Perez-Schofield J.B.G, 2009.
Efficient virtual machine support of runtime structural
reflection”, Science of Computer Programming, Vol.
74(10), pp. 836-860.
Ortin F., Zapico D., and Cueva J, 2007. Design patterns
for teaching type checking in a compiler construction
course. Education, IEEE Transactionson, 50(3):273–
283.
Ortin F., Zapico D., Perez-Schofield J.B.G., Garcia M.,
August 2010. Including both Static and Dynamic Typ-
ing in the same Programming Language. IET Soft-
ware, Volume 4, Issue 4, pp. 268-282.
Redondo J., Ortin F. and Cueva J, 2008. Optimizing Ref-
lective Primitives of Dynamic Languages. Internation-
al Journal of Software Engineering and Knowledge
Engineering, 18(6):759–783.
Torgersen M., 2004. The Expression Problem Revisited.
European Conference on Object-Oriented Program-
ming (ECOOP), pp. 123-143.
Wadler P., 1998. The expression problem, Posted on the
Java Genericity mailing list.
Watt D., and Brown D., 2000. Programming Language
Processors in Java: Compilers and Interpreters, Pren-
tice Hall.
A TYPE SAFE DESIGN TO ALLOW THE SEPARATION OF DIFFERENT RESPONSIBILITIES INTO PARALLEL
HIERARCHIES
25