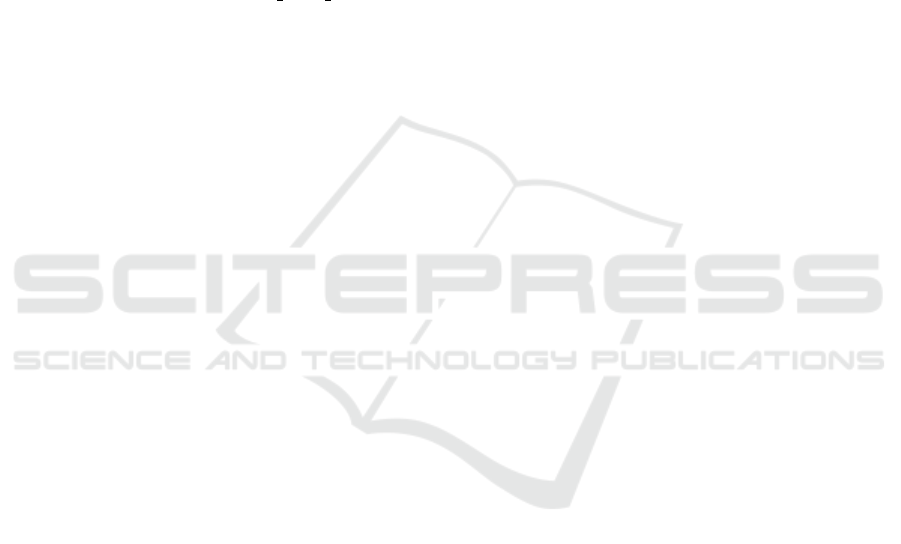
the @ACTIVITY annotation, with the body of the
method defining the computational behaviour of the
agent corresponding to the accomplishment of the ac-
tivity. Structured activities introduce the notion of
agenda to specify the hierarchical set of the potential
sub-activities composing the activity, referenced as
todo in the agenda. Each todo names the sub-activity
to execute, and optionally a pre-condition. When a
structured activity is executed, the todos in the agenda
are executed as soon as their pre-conditions hold, but
if no pre-condition is specified, the todo is immedi-
ately executed. Thus, multiple sub-activities can be
executed concurrently in the context of the same (su-
per) activity. A structured activity is implemented
by methods with an @ACTIVITY WITH AGENDA an-
notation, containing todo descriptions as a list of
@TODO annotations. A todo can be specified to be
persistent—in this case, once it has been completely
executed, it is reinserted in the agenda so as to be pos-
sibly executed again. This is useful to model cyclic
behaviour of agents when executing some activities.
In the A&A programming model, the artifact ab-
straction is useful to design passive resources and
tools that are used by agents as basic building blocks
of the environment. The functionality of an artifact
is structured in terms of operations whose execution
can be triggered by agents through artifact usage in-
terface.Similarly to the interface of objects or compo-
nents, the usage interface of an artifact defines a set
of controls that an agent can trigger so as to execute
operations, each one identified by a label and a list of
input parameters.Differently from the notion of ob-
ject interfaces, in this use interaction there is no con-
trol coupling: when an agent triggers the execution
of an operation, it retains its control (flow) and the
operation execution on the artifact is carried on inde-
pendently and asynchronously. The information flow
from the artifact to agents is modeled in terms of ob-
servable events generated by artifacts and perceived
by agents; therefore, artifact interface controls have
no return values. An artifact can also have some ob-
servable properties, allowing to inspect the dynamic
state of the artifact without necessarily executing op-
erations on it. Artifact templates in simpA can be cre-
ated by extending the base alice.simpa.Artifact
class. For example, an artifact representing a Web
page, storing its DOM model in an observable prop-
erty, and exposing an operation to change an attribute
of an element in the model, may be structured as fol-
lows:
public class Page extends Artifact {
@OBSPROPERTY Document dom;
@OPERATION void setAttribute(String id,
String attr,
String val) {
Element e = dom.getElementById(id);
e.setAttribute(attr, val);
updateProperty("DOM", dom);
}
}
Each operation listed in the artifact user interface is
defined as a method with an @OPERATION annotation.
Besides the usage interface, each artifact may define
a linking interface, applying the @LINK annotation on
operations that are meant to be invoked by other arti-
facts. Thus, it becomes possible to create complex
artifacts as a composition of simpler ones, assem-
bled dynamically by the linking mechanism. An ar-
tifact typically provides some level of observability,
either by generating observable events during execu-
tion of an operation, or by defining observable proper-
ties using the @OBSPROPERTY annotation. An observ-
able event can be perceived by the agent that has used
the artifact by triggering the operation generating the
event; changes to observable properties, triggered by
the updateProperty primitive, can be sensed by any
agent that has focussed on the artifact, without neces-
sarily having acted on it. Besides agents and artifacts,
the notion of workspace completes the basic set of ab-
stractions defined in A&A: a workspace is a logic con-
tainer of agents and artifacts, and it is the basic means
to give an explicit (logical) topology to the working
environment, so as to help scope the interaction inside
it. We conclude this section by focussing on the main
ingredients of the agent-artifact interaction model: a
more comprehensive account and discussion of these
and other features of agents, artifacts and workspaces
– outside the scope of this paper – can be found in
(Omicini et al., 2008; Ricci and Viroli, 2007).
2.1 Agent-Artifact Interaction: Use and
Observation
The interaction between agents and artifacts strictly
mimics the way in which people use their tools. For
a simple but effective analogy, let us consider a cof-
fee machine. The set of buttons of the coffee machine
represents the usage interface, while the displays that
are used to show the state of the machine represent
artifact observable properties. The signals emitted by
the coffee machine during its usage represent observ-
able events generated by the artifact.
Interaction takes place by means of a use action
(stage 1a in Figure 1, left), performed by an agent in
order to trigger the execution of an operation over an
artifact; such an action specifies the name and param-
eters of the interface control corresponding to the op-
eration. The observable events, possibly generated by
the artifact while executing an operation, are collected
AN AGENT-BASED PROGRAMMING MODEL FOR DEVELOPING CLIENT-SIDE CONCURRENT WEB 2.0
APPLICATIONS
15