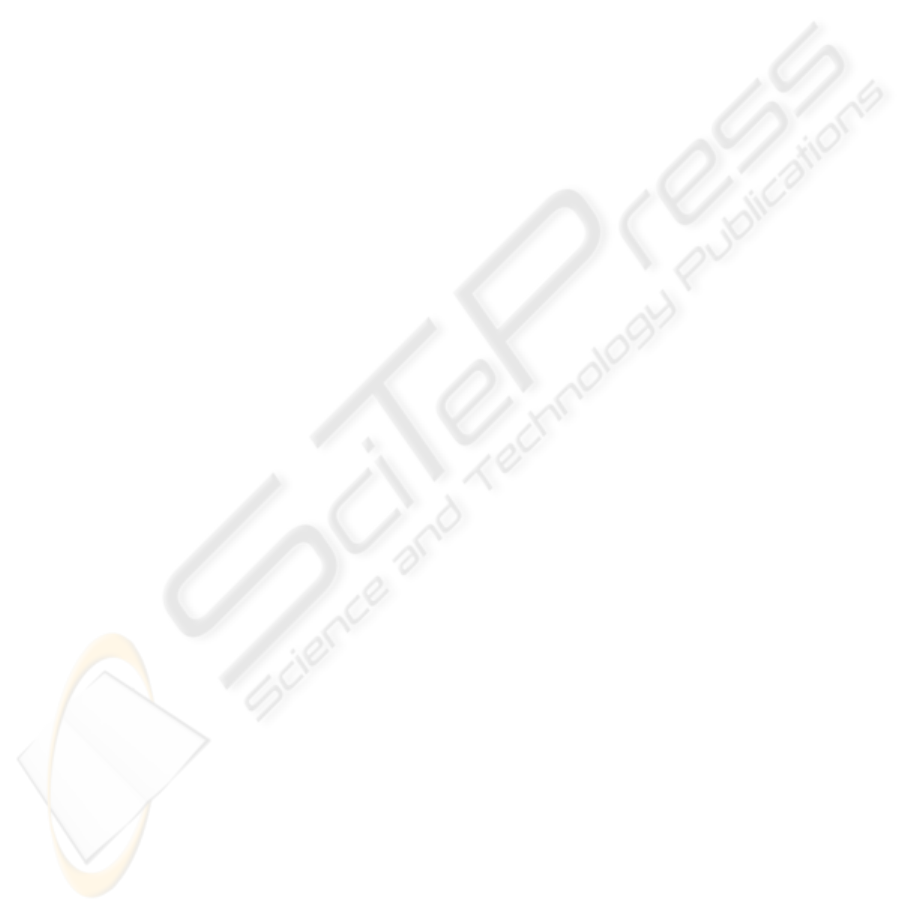
with AspectJ, leading to similar benefits as our
approach, namely reusability and better separation of
concerns.
6 CONCLUSIONS
In this paper we propose a programming technique
that enables expressing roles and collaborations. It is
lightweight in the sense that no changes to the under-
lying language are necessary. Instead, dynamic prox-
ies solve the problem of representing multiple objects
as one compound object. Our approach allows to
widen the set of membersof an objectat runtime, or in
other words, to dynamically augment its type. Never-
theless, user code can be statically type-checked. We
provide a Scala library as proof-of-concept and show
how extracting concerns into collaborations supports
reuse.
Future work will include applying our approach to
a larger project in order to verify its usefulness and
gain more insight about roles in programming lan-
guages. Another open task is a role-based library of
reusable implementationsof design patternsand other
recurring object collaborations. Moreover, other role
features should be investigated further, such as roles
restricting access to its core object and restrictions on
the sequence in which roles may be acquired and re-
linquished.
ACKNOWLEDGEMENTS
We would like to thank Prof. Uwe Aßmannfor inspir-
ing this work and the anonymous reviewers for their
comments and suggestions.
REFERENCES
B¨aumer, D., Riehle, D., Siberski, W., and Wulf, M. (1997).
The Role Object pattern. In Proceedings of the Con-
ference on Pattern Languages of Programs (PLoP
’97).
Gamma, E., Helm, R., Johnson, R., and Vlissides, J. (1995).
Design patterns: Elements of reusable object-oriented
software. Addison-Wesley Longman Publishing Co.,
Inc., Boston, MA, USA.
Guarino, N. (1992). Concepts, attributes and arbitrary re-
lations: some linguistic and ontological criteria for
structuring knowledge bases. Data Knowl. Eng.,
8(3):249–261.
Hannemann, J. and Kiczales, G. (2002). Design pattern
implementation in Java and AspectJ. SIGPLAN Not.,
37(11):161–173.
Harrison, W. (1997). Homepage on subject-
oriented programming and design patterns.
http://www.research.ibm.com/sop/sopcpats.htm.
Herrmann, S. (2007). A precise model for contextual roles:
The programming language ObjectTeams/Java. Ap-
plied Ontology, 2(2):181–207.
Herrmann, S., Hundt, C., and Mehner, K. (2004). Transla-
tion polymorphism in Object Teams. Technical report,
TU Berlin.
Kendall, E. A. (1999). Role model designs and implemen-
tations with aspect-oriented programming. SIGPLAN
Not., 34(10):353–369.
Kiczales, G., Lamping, J., Mendhekar, A., Maeda, C.,
Lopes, C. V., Loingtier, J.-M., and Irwin, J. (1997).
Aspect-oriented programming. In Proceedings of the
European Conference on Object-Oriented Program-
ming (ECOOP 1997), pages 220–242.
Kristensen, B. B. and Osterbye, K. (1996). Roles: concep-
tual abstraction theory and practical language issues.
Theor. Pract. Object Syst., 2(3):143–160.
Object Management Group OMG (2007). OMG Uni-
fied Modeling Language (OMG UML), superstruc-
ture, v2.1.2.
Odersky, M. (2008). Scala Language Spec-
ification. Version 2.7, http://www.scala-
lang.org/docu/files/ScalaReference.pdf.
Odersky, M., Spoon, L., and Venners, B. (2008). Program-
ming in Scala, A comprehensive step-by-step guide.
Artima.
Reenskaug, T., Wold, P., and Lehne, O. A. (1996). Work-
ing with Objects, The OOram Software Engineering
Method. Manning Publications Co.
Riehle, D. (1997). Composite design patterns. In OOPSLA
’97: Proceedings of the 12th ACM SIGPLAN confer-
ence on Object-oriented programming, systems, lan-
guages, and applications, pages 218–228, New York,
NY, USA. ACM Press.
Riehle, D. (2000). Framework Design: A Role Modeling
Approach. PhD thesis, ETH Z¨urich.
Smaragdakis, Y. and Batory, D. (2002). Mixin layers: an
object-oriented implementation technique for refine-
ments and collaboration-based designs. ACM Trans-
actions on Software Engineering and Methodology
(TOSEM), 11(2):215–255.
Steimann, F. (2000). On the representation of roles
in object-oriented and conceptual modelling. Data
Knowledge Engineering, 35(1):83–106.
ICSOFT 2008 - International Conference on Software and Data Technologies
20