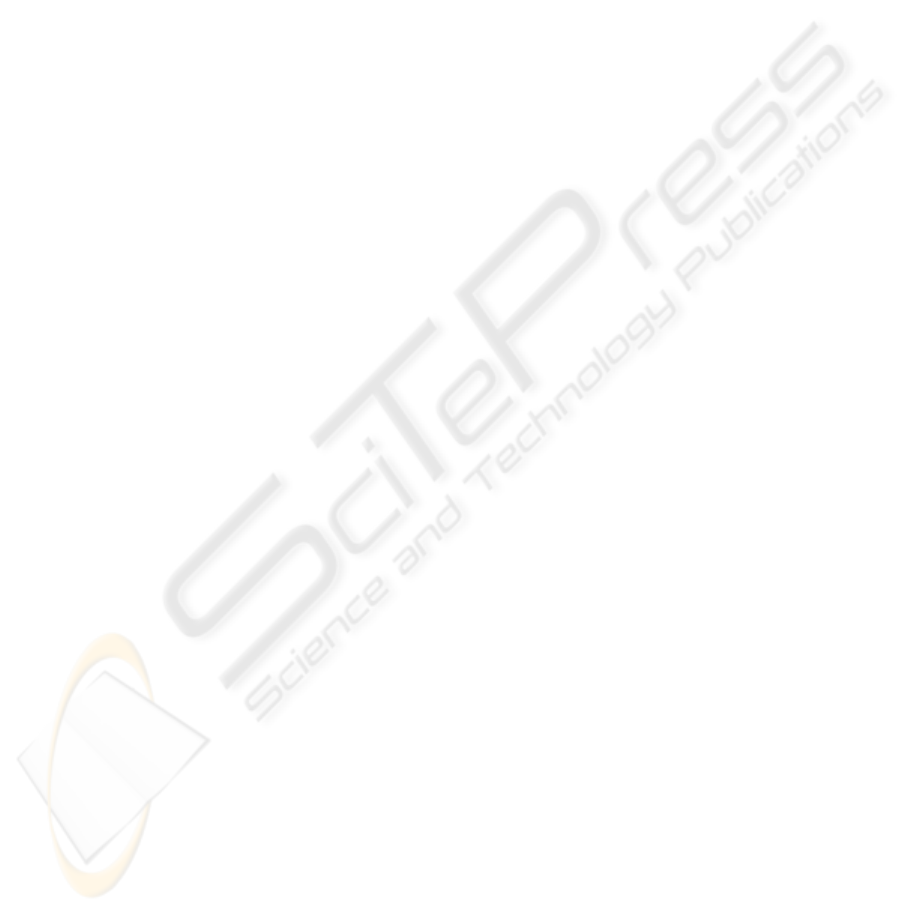
<Field type=’string’>French</Field>
<Field type=’string’>cheap</Field>
</TupleData>
</InputTuple>
Thirdly, while this is a simple demonstration, it
demonstrates the potential practical applicability of
eLindaWS. In particular, it would be a cost-effective
solution to this problem in the South African context,
where cellphone usage is far more widespread than
general Internet usage, and mobile data rates are con-
siderably cheaper than voice or SMS rates.
5 CONCLUSIONS
Our previous research has demonstrated both the de-
sirable simplicity and the improved performance that
can be obtained by extending traditional Linda sys-
tems with programmable matching facilities. The re-
search presented in this paper demonstrates that these
advantages can be extended to the field of distributed
web service applications.
The facility-locating midlet application demon-
strates that eLindaWS is suitable for use in mobile,
location-based applications, and illustrates the use of
a parameterized programmable matcher. While it
is only intended as a proof-of-concept prototype, it
functions in a realistic way, and could potentially be
the basis for a useful and cost-effective commercial
application, particularly in our South African context.
Future work to improve eLindaWS is likely to
concentrate on optimization, in particular:
• The optimization of the creation and parsing of
the XML documents (Ranganath et al., 2006)
• Improving the communication protocol used to
send the XML documents, especially for LAN
configurations
• Preprocessing the tuples before storing them in a
tuple space, e.g. indexing of the tuples, sorting of
the tuples, or other methods
ACKNOWLEDGEMENTS
This work was supported by the Distributed Mul-
timedia Center of Excellence in the Department of
Computer Science at Rhodes University, funded by
Telkom, Business Connexion, Comverse, Verso Tech-
nologies, StorTech, Tellabs, Amatole Telecommu-
nication Services, Mars Technologies, Bright Ideas
Projects 39 and THRIP. Financial support was also
received from the National Research Foundation.
REFERENCES
Carriero, N. and Gelernter, D. (1990). How to Write Paral-
lel Programs: A First Course. The MIT Press.
Fielding, R. and Taylor, R. (2002). Principled design of
the modern web architecture. ACM Trans. Internet
Technology, 2(2):115–150.
Freeman, E., Hupfer, S., and Arnold, K. (1999). JavaSpaces
Principles, Patterns, and Practice. Addison-Wesley.
Gelernter, D. (1985). Generative communication in Linda.
ACM Trans. Program. Lang. Syst., 7(1):80–112.
Lucchi, R. and Zavattaro, G. (2004). WSSecSpaces: a se-
cure data-driven coordination service for web services
applications. In SAC ’04: Proc. 2004 ACM Sym-
posium on Applied Computing, pages 487–491, New
York, NY, USA. ACM Press.
Mata, E.,
´
Alvarez, P., Ba˜nares, J., and Rubio, J. (2004). To-
wards an efficient rule-based coordination of web ser-
vices. In IBERAMIA 2004, volume 3315 of Lecture
Notes in Artificial Intelligence, pages 73–82. Springer
Verlag.
Mueller, B., Schul´e, L., and Wells, G. (2007). Using a tuple
space web service for parallel processing in bioinfor-
matics. In Proc. First Southern African Bioinformatics
Workshop, pages 34–37. Wits University.
Ranganath, V., King, A., and Andresen, D. (2006). Auto-
matic code generation for LYE, a high-performance
caching SOAP implementation. International Confer-
ence on Semantic Web and Web Services, Las Vegas.
Sun Microsystems (2006). Sun Java Wireless Toolkit.
http://java.sun.com/products/sjwtoolkit
.
Sun Microsystems (2007). Mobile Information Device Pro-
file (MIDP).
http://java.sun.com/products/-
midp
.
Wells, G. (2006). A tuple space web service for distributed
programming. In Arabnia, H., editor, Proc. Interna-
tional Conference on Parallel and Distributed Pro-
cessing Techniques and Applications (PDPTA’2006),
pages 444–450. CSREA Press.
Wells, G., Chalmers, A., and Clayton, P. (2004). Linda im-
plementations in Java for concurrent systems. Con-
currency and Computation: Practice and Experience,
16:1005–1022.
Wyckoff, P., McLaughry, S., Lehman, T., and Ford, D.
(1998). T Spaces. IBM Systems Journal, 37(3):454–
474.
Zenith, S. (1992). A rationale for programming with Ease.
In Banˆatre, J. and M´etayer, D. L., editors, Research
Directions in High-Level Parallel Programming Lan-
guages, volume 574 of Lecture Notes in Computer
Science, pages 147–156. Springer-Verlag.
WEBIST 2008 - International Conference on Web Information Systems and Technologies
100