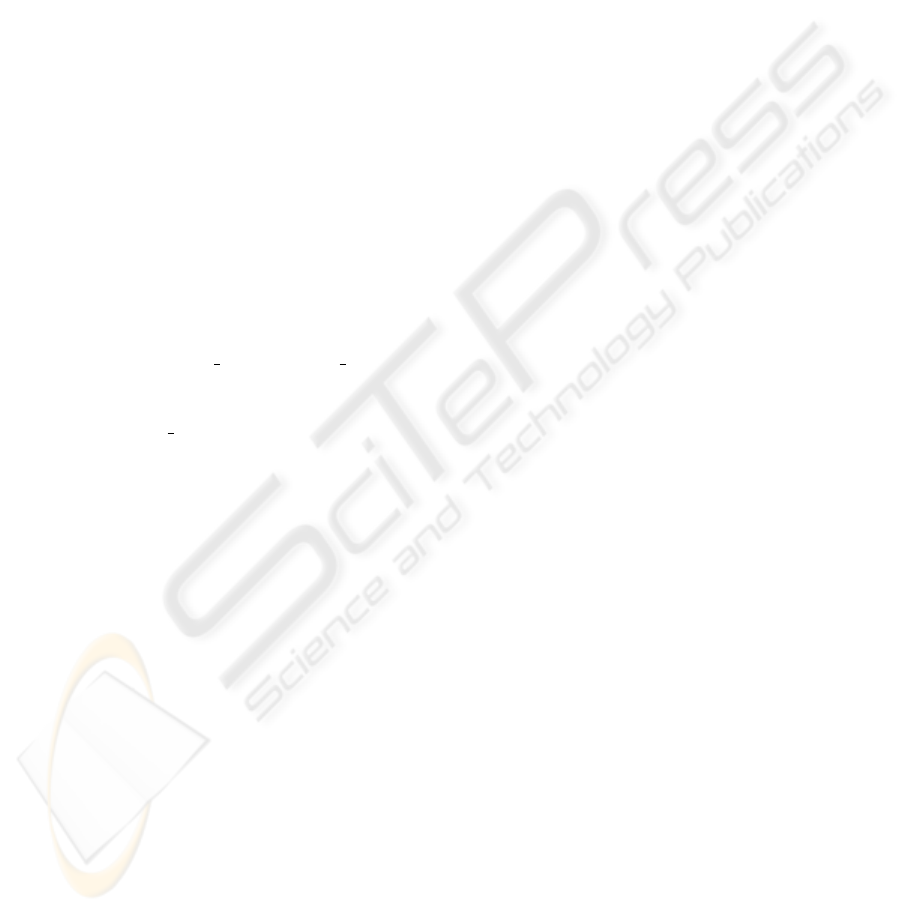
complex (mesh) and is therefore dimensionally and
topologically indepent. Only the relevant concepts,
in this case, the existence of edges incident to a ver-
tex and several quantity accessors, have to be met. In
other words, we have extended the concept program-
ming of the standard template library (STL) and the
generic programming of C++ to higher dimensional
data structures and automatic quantity access mecha-
nisms.
Compared to the reviewed related work given in
Section 2, our approach implements a domain spe-
cific embedded language. The related topological
concepts are given in Section 3, whereas Section 4
briefly overviews the used programming paradigms.
In Section 5 several application examples are pre-
sented. The first example introduces a problem of
a biological system with a simple PDE. The second
example shows a nonlinear system of coupled PDEs,
which makes use of the linearization framework intro-
duced in Section 4.1, where derivatives are calculated
automatically.
For a successful treatment of a domain specific
embedded notation several programming paradigms
are used. By object-oriented programming the ap-
propriate iterators are generated, hidden in this exam-
ple in the expression
vertex edge
and
edge vertex
.
Functional programming supplies the higher order
function expression between the
[
and
]
and the un-
named function object
1
. And finally the generic pro-
gramming paradigm (in C++ realized with parametric
polymorphism or templates) connects the various data
types of the iterators and quantity accessors.
A significant target of this work is the separa-
tion of data access and traversal by means of the
mathematical concept of fiber bundles (Butler and
Bryson, 1992). The related formal introduction en-
ables a clean separation of the internal combinatorial
properties of data structures and the mechanisms of
data access. A high degree of interoperability can be
achieved with this formal interface. Due to space con-
straints the performance analysis is omitted and we
refer to a recent work (Heinzl et al., 2006a) where the
overall high performance is presented in more detail.
2 RELATED WORK
In the following several related works are presented.
All of these software libraries are a great achievement
in the various fields of scientific computing.
The FEniCS project (Logg et al., 2003), which is
a unified framework for several tasks in the area of
scientific computing, is a great step towards generic
modules for scientific computing.
Femster (Castillo et al., 2005) is a class library
for finite element (FE) calculations. This means that
users must provide their own code for assembling
global FE matrices. In other words, Femster imple-
ments a general finite element API.
The Template Numerical Toolkit (Pozo, 1997)
is a collection of interfaces and reference implemen-
tations of numerical objects (matrices) in C++. The
toolkit defines interfaces for basic data structures,
such as multidimensional arrays and sparse matrices,
commonly used in numerical applications.
The Boost Graph Library is a generic interface
which enables access to a graph’s structure, but hides
the details of the actual implementation. All libraries
which implement this type of interface are interoper-
able with the BGL generic algorithms. This approach
was one of the first in the field of non-trivial data
structures with respect to interoperability. The prop-
erty map concept (Siek et al., 2002) was introduced
and heavily used.
The Grid Algorithms Library, GrAL (Berti,
2000) was one of the first contributions to the uni-
fication of data structures of arbitrary dimension for
the field of scientific computing. A common interface
for grids with a dimensionally and topologically inde-
pendent way of access and traversal was designed.
Our approach, the Generic Scientific Simulation
Environment, GSSE (Heinzl et al., 2006b) deals with
various modules for different discretization schemes
such as finite elements and finite differences. In
comparison, our approach focuses more on providing
building blocks for scientific computing, especially an
embedded domain language to express mathematical
dependencies directly, not only for finite elements.
To achieve interoperability between different li-
brary approaches we use concepts of the fiber bun-
dle theory to separate the base space and fiber space
properties. With this separation we can use several
other libraries (see Section 3) for different tasks. The
theory of fiber bundles separates the data structural
components from data access (fibers). We have de-
veloped a consistent data structure interface for all
different types of data structures and several other li-
braries employing the theory of CW-complexes and
poset theory. Based on this interface specification we
can use several libraries, such as STL, BGL, GrAL,
and accomplish high interoperability and code reuse.
3 CONCEPTS
Our approach extends the concept based program-
ming of the STL to arbitrary dimensions similar to
GrAL. The main difference to GrAL is the introduc-
MODERN CONCEPTS FOR HIGH-PERFOMANCE SCIENTIFIC COMPUTING - Library Centric Application Design
101