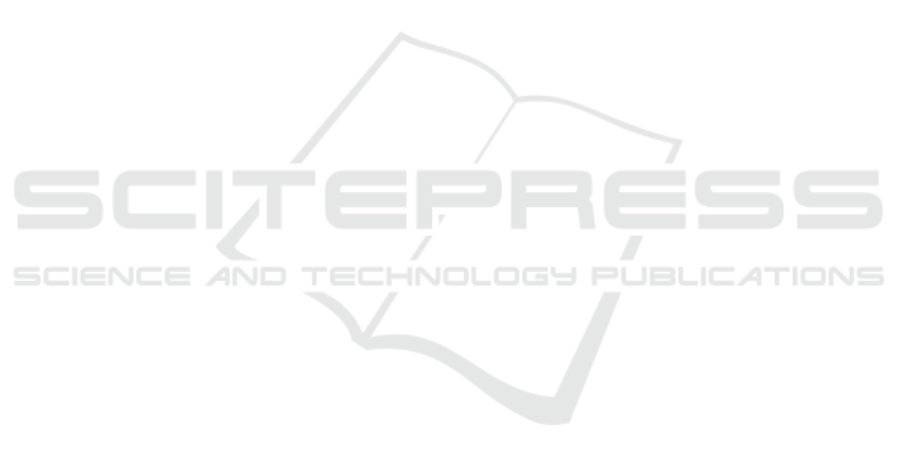
oped a framework, HeapHopper, based on bounded
model checking and framework execution, to analyze
the exploitability of different heap implementations.
Unlike these works, we propose a novel approach
for vulnerability detection. Although we also resort
to model checking, we propose its use differently. We
construct the stack memory state space of binary pro-
grams and use model checking to verify security prop-
erty violations over it.
8 CONCLUSIONS
In this paper, we introduced a model checking ap-
proach for binary programs, aimed at detecting stack
BO vulnerabilities by verifying security properties of
the stack memory. Our proposal includes developing
a theoretical framework for modelling stack memory
and formulating security properties for its analysis.
Future improvements should focus on increasing the
precision of state space generation to better identify
various stack BO vulnerabilities and expanding our
security properties to cover complex malicious behav-
iors such as return-oriented programming (ROP).
As the next steps, we plan to advance our model
by adding new security properties and enhancing LTL
formulas with additional predicates, aiming to im-
prove stack BO detection and categorization. We will
then evaluate our approach with diverse binaries of
C applications, focusing on the precision in vulner-
ability detection and the scalability of our approach.
Based on the evaluation outcomes, we may adjust the
model and properties to enhance performance.
ACKNOWLEDGMENTS
This work was supported by FCT through the
LASIGE Research Unit, ref. UIDB/00408/2020
(https://doi.org/10.54499/UIDB/00408/2020) and ref.
UIDP/00408/2020 (https://doi.org/10.54499/UIDP/
00408/2020)
REFERENCES
Butt, M. A., Ajmal, Z., Khan, Z. I., Idrees, M., and Javed, Y.
(2022). An in-depth survey of bypassing buffer over-
flow mitigation techniques. Applied Sciences, 12(13).
Chen, H. and Wagner, D. (2002). Mops: an infrastructure
for examining security properties of software. Pro-
ceedings of the ACM Conference on Computer and
Communications Security.
Choi, J., Jang, J., Han, C., and Cha, S. K. (2019). Grey-box
concolic testing on binary code. In IEEE/ACM Inter-
national Conference on Software Engineering (ICSE),
pages 736–747.
Clarke, E. M., Henzinger, T. A., Veith, H., and Bloem,
R., editors (2017). Handbook of Model Checking.
Springer Cham.
Eckert, M., Bianchi, A., Wang, R., Shoshitaishvili, Y.,
Kruegel, C., and Vigna, G. (2018). Heaphopper:
bringing bounded model checking to heap implemen-
tation security. In Proceedings of the USENIX Secu-
rity Symposium, page 99–116.
Eilam, E. (2005). Reversing: Secrets of Reverse Engineer-
ing. John Wiley & Sons, Inc., USA.
Gastin, P. and Oddoux, D. (2001). Fast ltl to b
¨
uchi automata
translation. In Comp. Aided Verification, pages 53–65.
Huang, Y.-W., Yu, F., Hang, C., Tsai, C.-H., Lee, D., and
Kuo, S.-Y. (2004). Verifying web applications using
bounded model checking. In International Conference
on Dependable Systems and Networks, 2004, pages
199 – 208.
In
´
acio, J. and Medeiros, I. (2023). Corca: An automatic
program repair tool for checking and removing effec-
tively c flaws. In IEEE Conference on Software Test-
ing, Verification and Validation (ICST), pages 71–82.
Kaur, A. and Nayyar, R. (2020). A comparative study of
static code analysis tools for vulnerability detection
in c/c++ and java source code. Procedia Computer
Science, 171:2023–2029.
Kroening, D. and Tautschnig, M. (2014). Cbmc – c bounded
model checker. In Tools and Algorithms for the
Construction and Analysis of Systems, volume 8413,
pages 389–391.
Kroes, T., Koning, K., Kouwe, E., Bos, H., and Giuffrida, C.
(2018). Delta pointers: buffer overflow checks with-
out the checks. In Proceedings of the Thirteenth Eu-
roSys Conference, pages 1–14.
Li, J., Zhao, B., and Zhang, C. (2018). Fuzzing: a survey.
Cybersecurity, 1.
Mercer, E. and Jones, M. (2005). Model checking ma-
chine code with the gnu debugger. In Proceedings of
the 12th International Conference on Model Checking
Software, page 251–265.
Nadeem, M., Williams, B. J., and Allen, E. B. (2012). High
false positive detection of security vulnerabilities: a
case study. In Proceedings of the 50th Annual South-
east Regional Conference, page 359–360.
Nguyen, H.-V. and Touili, T. (2017). Caret model check-
ing for malware detection. In Proceedings of the
24th ACM SIGSOFT International SPIN Symposium
on Model Checking of Software, page 152–161.
One, A. (1996). Smashing the stack for fun and profit.
Phrack, 7(49).
Sharma, T., Kechagia, M., Georgiou, S., Tiwari, R., Vats,
I., Moazen, H., and Sarro, F. (2024). A survey on
machine learning techniques applied to source code.
Journal of Systems and Software, 209:111934.
Vadayath, J., Eckert, M., Zeng, K., Weideman, N., Menon,
G., Fratantonio, Y., Balzarotti, D., Doup
´
e, A., Bao, T.,
Wang, R., Hauser, C., and Shoshitaishvili, Y. (2022).
Arbiter: Bridging the static and dynamic divide in vul-
nerability discovery on binary programs. In Proc. of
the USENIX Security Symposium, pages 413–430.
ENASE 2024 - 19th International Conference on Evaluation of Novel Approaches to Software Engineering
726