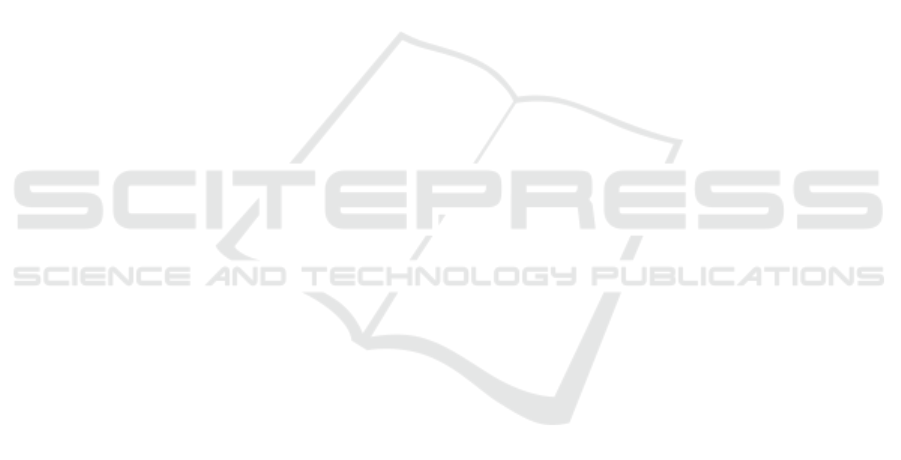
polygon resulting from the previous step can be trian-
gulated in O(k) time by iterating over the vertices in
decreasing y-direction and connecting the left and the
right chain. The overall worst-case runtime of the tri-
angulation step is thus in O(k log k). Again, the num-
ber k of input vertices equals the number n of contour
edges, so that the runtime is in O(nlogn).
Since the orientation of the original edges is re-
tained during triangulation, the cap mesh is consis-
tently orientated with the clipped mesh. If the trian-
gulation is not consistently oriented in itself, this can
easily be corrected using an O(n) scan over the list
of triangles. Moreover, a simple runtime optimization
of the triangulation can be added by testing each poly-
gon for convexity and applying a very simple O(n) tri-
angulation for convex polygons instead of the afore-
mentioned algorithm. However, this does not change
the upper bound of the runtime complexity.
For the set of triangles resulting from the trian-
gulation, the original 3D positions of the vertices (as
computed during clipping) are used instead of the ver-
tex positions transformed into the xy-plane. If the
vertices contain normal vectors, the normal vector at-
tribute for all of the vertices in the cap mesh is set to
the inverted normal of the clipping plane, which cor-
responds to the normal vector of the cut surface. After
finishing the triangulation step for all polygons, the
cap mesh is complete. The output geometry is then
created by combining the cap mesh with the clipped
input mesh (e.g., by uploading the cap mesh to the
GPU). Since each contour edge of the cap mesh is
shared between a triangle of the clipped input mesh
and a triangle of the cap mesh, and the shared edges
induce opposite direction in the meshes due to the
output of the clipping step, the output mesh is a con-
sistently oriented closed two-manifold triangle mesh.
Since the runtime of the clipping step (if not per-
formed in parallel) is O(N) and each subsequent step
of the algorithm is in O(n logn) where n is the num-
ber of triangles intersecting the plane, the algorithm
has an overall worst case runtime of O(N + nlog n).
5 CONCLUSION AND FUTURE
WORK
We have proposed an efficient method for geometri-
cally clipping and capping a closed two-manifold tri-
angle mesh in O(N + n logn). Our method performs
the clipping on the GPU and transfers the contour
edges to the CPU, where the cap mesh is computed to
close the clipped input mesh. One of the drawbacks of
our algorithm is the numerical stability, which might
be problematic particularly in the loop construction.
However, this can be mitigated by using a small ep-
silon as an allowed distance between the end vertex of
an edge and the start vertex of its successor. Another
problem of our method may be the quality of the tri-
angulation in the last step. In future work, we will try
to improve this step by applying constrained Delau-
nay triangulation or use of additional Steiner vertices
to increase the quality of the cap mesh.
REFERENCES
Bajaj, C. L. and Dey, T. K. (1990). Polygon nesting and
robustness. Inf. Proc. Lett., 35(1):23–32.
Burns, M. and Finkelstein, A. (2008). Adaptive cutaways
for comprehensible rendering of polygonal scenes.
ACM Transactions on Graphics, 27(5):154:1–154:7.
Chazelle, B. (1991). Triangulating a simple polygon in lin-
ear time. Discrete & Comput. Geom., 6(5):485–524.
de Berg, M., Cheong, O., van Kreveld, M., and Overmars,
M. (2008). Computational Geometry: Algorithms and
Applications. Springer, 3rd edition.
Erleben, K. and Henriksen, K. (2006). A simple plane
patcher algorithm. Technical Report DIKU-TR-
06/09, Department of Computer Science, University
of Copenhagen.
Foley, J. D., van Dam, A., Feiner, S. K., and Hughes, J. F.
(1996). Computer Graphics: Principles and Practice,
2nd ed. in C. Addison-Wesley.
Garey, M. R., Johnson, D. S., Preparata, F. P., and Tarjan,
R. E. (1978). Triangulating a simple polygon. Inf.
Proc. Let., 7(4):175–179.
Huang, J., Yagel, R., Filippov, V., and Kurzion, Y. (1998).
An accurate method for voxelizing polygon meshes.
In Proceedings of the 1998 IEEE Symposium on Vol-
ume Visualization, VVS ’98, pages 119–126. ACM.
Lee, D. T. and Preparata, F. P. (1977). Location of a point
in a planar subdivision and its applications. SIAM J.
on Computing, 6(3):594–606.
Lewiner, T., Lopes, H., Vieira, A. W., and Tavares, G.
(2003). Efficient implementation of marching cubes’
cases with topological guarantees. J. of Graphics
Tools, 8:2003.
McGuire, M. (2011). Efficient triangle and quadrilateral
clipping within shaders. J. of Graphics, GPU, and
Game Tools, 15(4):216–224.
McReynolds, T. and Blythe, D. (2005). Advanced Graphics
Programming Using OpenGL. Morgan Kaufmann.
Schwarz, M. and Seidel, H.-P. (2010). Fast parallel surface
and solid voxelization on gpus. ACM Transactions on
Graphics, 29(6):179:1–179:10.
Sutherland, I. E. and Hodgman, G. W. (1974). Reentrant
polygon clipping. Comm. of the ACM, 17(1):32–42.
Trapp, M. and D
¨
ollner, J. (2013). 2.5d clip-surfaces for
technical visualization. J. of WSCG, 21(1):89–96.
Weiskopf, D., Engel, K., and Ertl, T. (2003). Interactive
clipping techniques for texture-based volume visual-
ization and volume shading. IEEE Transactions on
Visualization and Computer Graphics, 9(3):298–312.
GRAPP 2017 - International Conference on Computer Graphics Theory and Applications
194