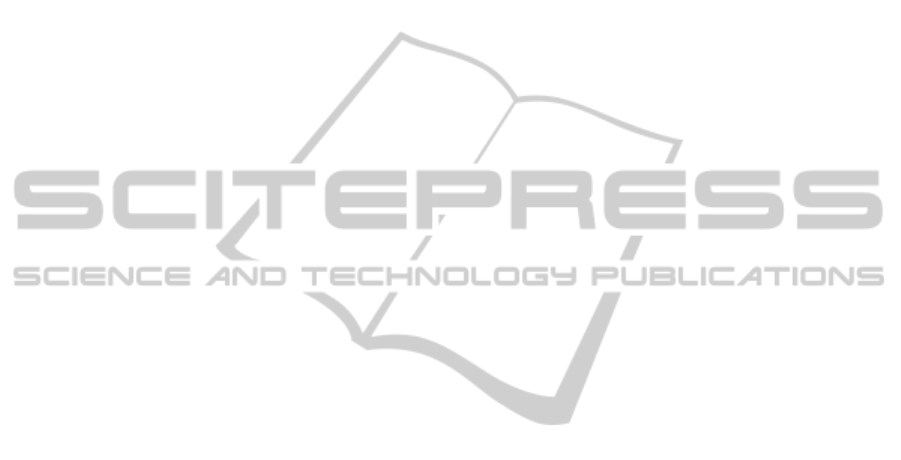
6 FUTURE WORK
Given the strong encapsulation exhibited by Insense
components, as well as the low coupling between
them, an obvious next step is to include dynamic re-
programming via the radio in Insense and an imple-
mentation in InceOS. This will enable dynamic, over-
the-air composition of components at runtime.
Currently the radio interaction is exposed to the
programmer and is the only means of inter-node com-
munication. Although the actual hardware interaction
is abstracted, the programmer is aware of the radio
transfer via the Radio component. Future work will
include the ability to introspect and react to the nodes
network environment, thus making it possible to dis-
cover a node’s neighbours and be alerted to changes.
New language-based systems can only become
prevalent if sufficient program development, testing,
verification, deployment, and runtime monitoring ca-
pabilities are also provided. We are building such a
development environment for Insense/InceOS-based
systems.
7 CONCLUSIONS
The language-specific operating system, InceOS, en-
ables programs written in Insense to exhibit the space
and time efficiency needed for production use in sen-
sor networks. A comparison of Insense/InceOS and
nesC/TinyOS code for a range of applications shows
that it is possible to write functionally-equivalent pro-
grams in fewer lines of Insense and that InceOS appli-
cations consistently outperform their TinyOS counter-
parts. Thus, use of the component-based abstractions
provided by Insense coupled with the efficient sup-
port for these abstractions in InceOS facilitates the
development of WSN applications that exhibit state-
of-the-art performance while reducing programming
complexity.
More generally, we conclude that provision of
a language-specific operating system is an effective
mechanism for making programs written in higher-
level languages competitive with equivalent programs
written in lower-level languages supported by more
general-purpose operating systems.
REFERENCES
Burrell, J., Brooke, T., and Beckwith, R. (2004). Vineyard
computing: Sensor networks in agricultural produc-
tion. IEEE Pervasive Computing, 3:38–45.
Dearle, A., Balasubramaniam, D., Lewis, J., and Morri-
son, R. (2008). A component-based model and lan-
guage for wireless sensor network applications. In
COMPSAC ’08: Proceedings of the 2008 32nd An-
nual IEEE International Computer Software and Ap-
plications Conference, pages 1303–1308, Washing-
ton, DC, USA. IEEE Computer Society.
Dunkels, A., Gronvall, B., and Voigt, T. (2004). Contiki
- a lightweight and flexible operating system for tiny
networked sensors. In LCN ’04: Proceedings of the
29th Annual IEEE International Conference on Lo-
cal Computer Networks, pages 455–462, Washington,
DC, USA. IEEE Computer Society.
Gay, D., Levis, P., von Behren, R., Welsh, M., Brewer, E.,
and Culler, D. (2003). The nesC language: A holistic
approach to networked embedded systems. In PLDI
’03: Proceedings of the ACM SIGPLAN 2003 con-
ference on Programming language design and imple-
mentation, volume 38, pages 1–11, New York, NY,
USA. ACM.
Hasler, A., Talzi, I., Tschudin, C., and Gruber, S. (2008).
Wireless sensor networks in permafrost research
- concept, requirements, implementation and chal-
lenges. In Proc. 9th Intl Conf. on Permafrost (NICOP
2008.
Hauer, J. (2006). nesc sense application reposi-
tory. Web Site. http://code.google.com/p/tinyos-
main/source/browse/trunk/apps/Sense/?r=2898 , Ac-
cessed on 25/09/2011.
Hewitt, C., Bishop, P., and Steiger, R. (1973). A universal
modular actor formalism for artificial intelligence. In
Proceedings of the 3rd international joint conference
on Artificial intelligence, pages 235–245, San Fran-
cisco, CA, USA. Morgan Kaufmann Publishers Inc.
Hill, J., Szewczyk, R., Woo, A., Hollar, S., Culler, D.,
and Pister, K. (2000). System architecture directions
for networked sensors. SIGOPS Oper. Syst. Rev.,
34(5):93–104.
Klues, K., Liang, C.-J. M., Paek, J., Mus
˘
aloiu-E, R., Levis,
P., Terzis, A., and Govindan, R. (2009). Tosthreads:
thread-safe and non-invasive preemption in tinyos. In
Proceedings of the 7th ACM Conference on Embedded
Networked Sensor Systems, SenSys ’09, pages 127–
140, New York, NY, USA. ACM.
Milner, R., Parrow, J., and Walker, D. (1992). A calculus of
mobile processes, i. Inf. Comput., 100(1):1–40.
Porter, B. and Coulson, G. (2009). Lorien: a pure dy-
namic component-based operating system for wire-
less sensor networks. In MidSens ’09: Proceedings of
the 4th International Workshop on Middleware Tools,
Services and Run-Time Support for Sensor Networks,
pages 7–12, New York, NY, USA. ACM.
Rondini, E. and Hailes, S. (2007). Distributed compu-
tation in wireless ad hoc grids with bandwidth con-
trol. In Proceedings of the 5th international confer-
ence on Embedded networked sensor systems, SenSys
’07, pages 437–438, New York, NY, USA. ACM.
SENSORNETS 2012 - International Conference on Sensor Networks
44