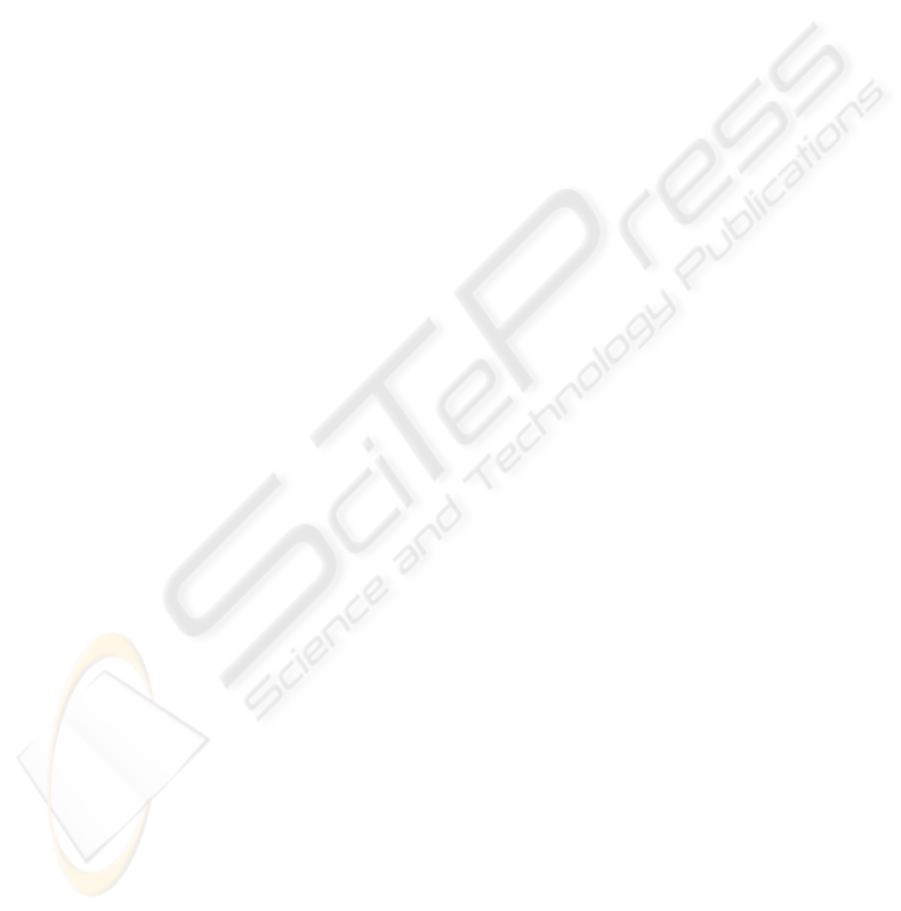
2 RELATED WORK
The use of simulations and animations to increase
understanding of fundamental principles is of course
new new and has been used for decades. As far back
as the 1970’s the Control Data Corporation PLATO
system (Control Data Corporation and PLATO Learn-
ing Inc., 2009) made extensive use of graphics, an-
imation, and simulation to help teach undergradu-
ate and graduate students. However, the use of the
PLATO in educational settings was primarily used for
“drills” with customized feedback to the users, rather
than being used in the classroom. One notable excep-
tion was the CDC Assembly Language Simulator for
PLATO, that allowed users to write, execute, and de-
bug programs both in the CDC CPU language and the
CDC PPU language.
More recently, Jerding et. al (Jerding et al., 1997)
have discussed using visualization to observe the flow
of a given program, primarily to assist in debug-
ging and performance analysis. Earlier, Stasko et.
al (Stasko, 1991) designed the Tango system, that pro-
vides a simple and easy-to-use interface for instruc-
tors to create animations of algorithm execution. The
Tango system produced animations similar to our par-
allel bubble sort animation discussed later.
Carothers et. al (Carothers et al., 1997) created a
visualization environment that we used to observe the
execution of a parallel and distributed discrete event
simulation environment. Their PvaniM system was
primarily intended to allow a user to observe possible
performance bottlenecks in an optimistic distributed
simulation, and to compare various middleware ap-
proaches to obtain optimal performance.
The commonly used SPIM (Larus, 2009) MIPS
simulation tool is widely used to help students design,
implement, and debug assembly language programs.
While the SPIM environment does have a comprehen-
sive and friendly graphical user interface, it does not
animate the actual execution steps within an instruc-
tion (such as computing the effective memory address
and fetching operands from memory).
The preceding is only a small sampling of a large
number of computer–based simulations that have
been frequently used to provide detailed insight into
computer and computer program operation. We have
developed simulations and animations that are specifi-
cally designed to help students understand fundamen-
tal concepts in computer architecture, algorithms, and
networking.
3 ANIMATION DESCRIPTIONS
This section describes in detail several of the simu-
lation and animation tools we have created recently.
We have focused our design and features specifically
for use by classroom instructors during the lectures, to
give students additional visual clues for the individual
concept being presented.
3.1 CPU Animations
Given the current popularity of multi–core architec-
tures, such as the Intel Core 2 Duo, we decided that
an introduction to parallel computation was needed
very early in the Electrical Engineering curriculum.
In order to provide a deep understanding of the is-
sues in parallel programs, such as race conditions, in-
terlocks, deadlocks and livelocks, we decided to in-
troduce students to lower–level assembly language in
the first weeks of CS1372. Our objective was not to
teach the students any individual assembly language
such as Intel i386, but rather teach the fundamental
concepts of memory accesses, register to register op-
erations, and branching instructions. Without this de-
tailed understanding of what is happening “under the
covers”, it is hard for students to realize the conse-
quences of seemingly innocuous instructions like “i
= i + 1” in a multi–processing environment. In or-
der to illustrate these assembly language principles,
we developed the CompSim program.
Since we were not interested in any one particu-
lar machine language, we decided the simplest and
easiest approach was to define our own assembly lan-
guage. We included the usual arithmetic instructions,
load and store instructions (with both “load from im-
mediate” and “load from memory”), and the usual
conditional and unconditional branching instructions.
Even though we only supported 20 instructions, they
are sufficiently capable to design and implement any
arbitrarily complex program. The key feature of
CompSim was of course the animation output.
First we will discuss and show the Single CPU
simulation supported by CompSim. A snapshot of
the initial state of CompSim is shown in figure 1(a).
Shown clearly are the CPU components (Instruction
Register, Program Counter, and the eight Registers),
the data memory, and the instruction memory. The
question mark characters shown in several of the reg-
isters indicates an uninitialized value. This particular
“program” calculates the sum and average of an ar-
ray of numbers, with the length of the array found in
a specific memory location. Next, figure 1(b) shows
the instruction fetch cycle, which reads the next in-
struction at the address specified by the PC register,
CSEDU 2010 - 2nd International Conference on Computer Supported Education
474