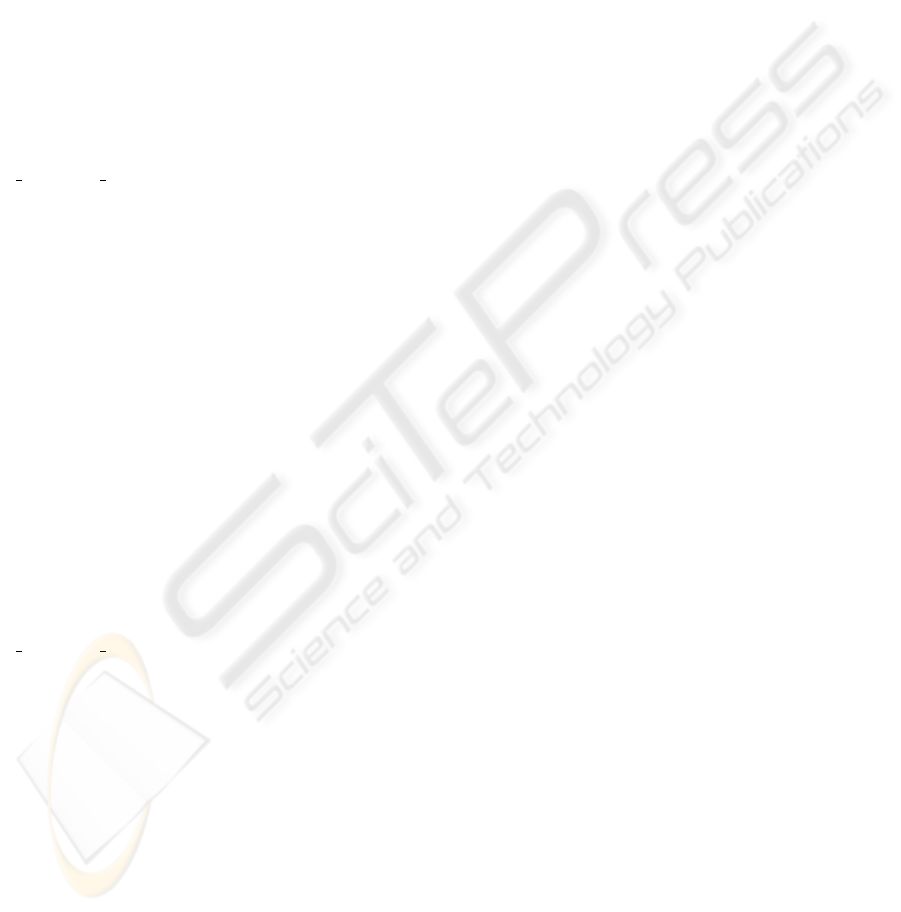
angle fan methods. 3) The grid test, one of the fastest
methods, which is based on a regular space decompo-
sition.
To make the comparison we have used the imple-
mentation of Eric Haines (Haines, 1994) in Graphics
Gems IV. The tests consist in 1000 consecutive point
tests performed on 5 different random polygons, with
sizes from 10 to 10000 edges. The target hardware
consists of a Intel Pentium IV processor at 1.6 GHz,
with 512 MB of RAM memory. The graphics card
is an NVIDIA GeForce 6600. In the tests based on
graphics hardware the size of the P-buffer where the
polygon is drawn is 400x400. Table 1 shows the exe-
cution times of the different tests.
As it can be seen, the results obtained by the
approaches based on the occlusion query mecha-
nism are very good. The approach based on the
NV
occlusion query extension beats the crossings
and Spackman methods for polygon from 100 edges.
Next, we describe the main advantages of the tests
presented in the paper based on graphics hardware:
• Their execution times are almost constant, they do
not depend on the number of vertices of the poly-
gon.
• The tests, as well as their preprocessings, are
very simple, easy to understand, and they have an
straightforward implementation.
• They work with the GPU memory, saving RAM
memory.
• As “alternative data structure” an off-screen
buffer is used, whose size is constant, not depend-
ing on the size of the polygon.
• They work with any kind of polygon: concave,
with holes, with intersecting edges, etc.
Furthermore, the test based on the
NV
occlusion query extension has the advan-
tage that multiple queries can be done in parallel with
CPU execution.
And next we describe the main drawbacks of these
tests:
• As other methods they need preprocessing —to
draw the polygon—. Therefore, they are mainly
suitable when a high number of tests is needed.
Table 2 compares the preprocessing times of the
different tests.
• The precision of the tests depends on the resolu-
tion of the P-buffer, and it is lower than software
based tests.
Finally, another drawback of the tests based on the
occlusion query mechanism is that they cannot be ex-
tended to solve picking.
6 CONCLUSION
The occlusion query extensions of modern GPUs
were originally intended for supporting occlusion
culling algorithms. This paper has shown that these
extensions can also be used to develop two original,
efficient point-in-polygon tests. The tests, as well as
their preprocessings, are easy to understand and they
have a straightforward implementation. Besides, they
work with any kind of polygon, they are fast and their
execution times do not depend on the number of edges
of the polygon. Finally, one of the tests allows mul-
tiple point-in-polygon queries to be done in parallel
with CPU execution.
However, the tests have some drawbacks: their
precision is lower than software based tests, they need
preprocessing, and they cannot be extended to solve
picking.
ACKNOWLEDGEMENTS
This work has been partially granted by the Ministe-
rio de Ciencia y Tecnolog
´
ıa of Spain and the Euro-
pean Union by means of the ERDF funds, under the
research project TIN2004-06326-C03-03 and by the
Conserjer
´
ıa de Innovaci
´
on, Ciencia y Empresa of the
Junta de Andaluc
´
ıa, under the research project P06-
TIC-01403.
REFERENCES
A. Rueda, R. Segura, F. F. and Ruiz, J. (2004). Rasterizing
complex polygons without tesselations. In Graphical
Models. 66(3) 127-132.
Akenine-Moller, T. and Haines, E. (2002). Real–Time Ren-
dering. A.K. Peters, Massachusetts, 2nd edition.
Antonio, F. (1992). Faster line segment intersection.
David Kirk (Ed.) Graphics Gems III Academic Press,
Boston, 1st edition.
Hacker, R. (1962). Certification of algorithm 112: position
of point relative to polygon. In Communications of the
ACM. Vol. 5 pp. 606.
Haines, E. (1994). Point in polygon strategies. Paul Heckert
(Ed.) Graphics Gems IV Academic Press, New York,
1st edition.
Hanrahan, P. and Haeberli, P. (1990). Direct wysiwyg paint-
ing and texturing on 3d shapes. In Computer Graphics
(SIGGRAPH ’90 Proceedings). 24(4) 215-223.
Shimrat, M. (1962). Algorithm 112: position of point rela-
tive to polygon. In Communications of the ACM. Vol.
5 pp. 434.
Spackman, J. (1993). Simple, fast triangle intersection, part
ii. In Ray Tracing News. 6(2).
GRAPP 2007 - International Conference on Computer Graphics Theory and Applications
164